Bash, the magic that empowers the Cloud
Bash, a popular command-line shell and scripting language used in Unix-like operating systems - Short Intro & related tools
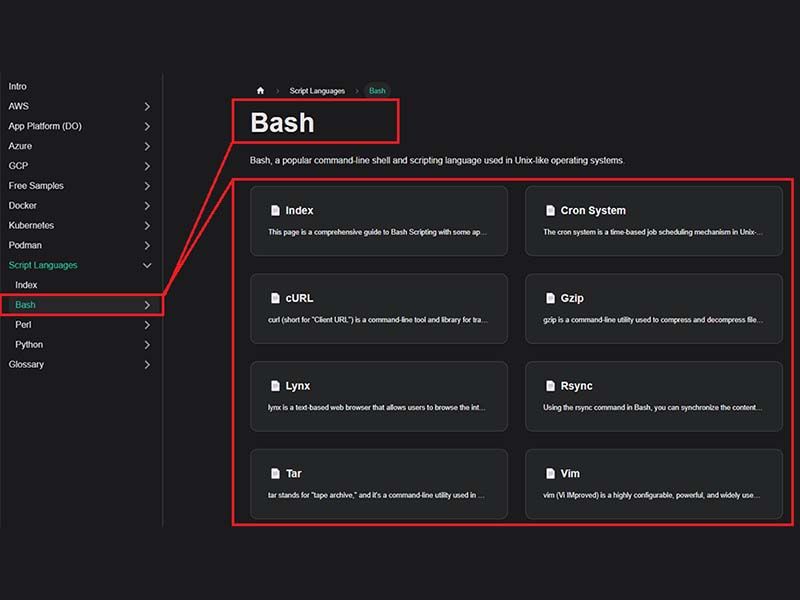
Hello! This article is not about ML, Generative AI, automation, or any other hot topic. It’s about Bash, also known as `Bourne Again Shell` the workhorse that empowers Cloud Computing and Services like AWS, GCP, Azure or Digital Ocean.
Content provided by DeployPRO — Deployment process simplified
With decent Bash knowledge, anyone can manage a Linux system with ease, deploy projects LIVE, schedule backups, and execute maintenance tasks using the CRON System. If this sounds like something useful, let’s move forward and point out a few Bash-related topics and tools.
What is Bash?
Bash, short for “Bourne Again Shell” is a command-line interface and scripting language used in Unix-like operating systems. It provides a way to interact with the operating system by running commands, and it also supports writing scripts to automate tasks.
Getting Started
- Open a terminal on your Linux system. This is where you’ll interact with Bash.
- Type a command and press Enter to execute it. For example,
ls
lists files in the current directory. - Arguments can be added after the command, like
ls -l
to list files in long format.
Basic Commands
ls
: List files and directories in the current location.cd
: Change directory.pwd
: Print the current working directory.mkdir
: Create a new directory.touch
: Create an empty file.cp
: Copy files or directories.mv
: Move or rename files or directories.rm
: Remove files or directories (use with caution).
Variables
Bash uses variables to store and manipulate data.
name="John"
echo "Hello, $name!"
Input and Output
echo
: Print text to the screen.read
: Read input from the user.
echo "What's your name?"
read name
echo "Hello, $name!"
Conditional Statements
Bash supports if
statements for conditional execution.
age=25
if [ "$age" -lt 18 ]; then
echo "You're a minor."
else
echo "You're an adult."
fi
Loops
for fruit in apple banana orange; do
echo "I like $fruit."
done
Functions
greet() {
echo "Hello, $1!"
}
greet "Alice"
Simple Calculator Script
#!/bin/bash
read -p "Enter first number: " num1
read -p "Enter second number: " num2
sum=$((num1 + num2))
echo "Sum: $sum"
Bash & Linux Tools
This section presents a few popular tools we can use to manage different aspects of a Linux box like backups, text browsing, and file editing.
Cron System
The cron system is a time-based job scheduling mechanism in Unix-like operating systems. It allows you to automate repetitive tasks by scheduling them to run at specific intervals. The term “cron” comes from the Greek word “chronos,” meaning time.
Components of the Cron System
- Cron Daemon (
cron
): The cron daemon is a background service that manages the scheduling and execution of cron jobs. It constantly checks the system's crontab files to determine when to run scheduled tasks. - Cron Jobs: A cron job is a command or script that you want to run automatically at a specified time or interval. Each cron job consists of a command and a schedule defined by cron syntax.
Cron Syntax: Cron uses a specific syntax to define when a job should be executed. The syntax consists of five fields (plus an optional command field):
* * * * * command_to_run
| | | | |
| | | | +----- Day of the week (0 - 6) (Sunday = 0)
| | | +------- Month (1 - 12)
| | +--------- Day of the month (1 - 31)
| +----------- Hour (0 - 23)
+------------- Minute (0 - 59)
Cron Samples
- Run a Script Every Night at Midnight
0 0 * * * /path/to/script.sh
- Send System Statistics Every Hour
0 * * * * /usr/bin/collect_stats.sh
cURL — Client URL
curl
(short for "Client URL") is a command-line tool and library for transferring data with URLs. It supports various protocols, including HTTP, HTTPS, FTP, FTPS, SCP, SFTP, LDAP, and more. curl
is widely used to interact with web services, download files, and perform data transfers from the command line in Unix-like operating systems.
cURL Samples
- Downloading a File
curl -O https://www.example.com/file.txt
- Fetching Only Headers
curl -I https://www.example.com
- Sending POST Data
curl -X POST -d "key=value" https://www.example.com/post_endpoint
curl
is a powerful and flexible tool for interacting with web resources from the command line. Its wide range of features and support for various protocols make it a valuable tool for both casual users and developers.
Gzip
gzip
is a command-line utility used to compress and decompress files in Unix-like operating systems. It is named after the "GNU zip" project, which aims to provide a free and efficient replacement for the older compress
utility.
Basic Usage
gzip [options] file
Gzip Samples
- Compressing a File
gzip file.txt
- Decompressing a File
gzip -d file.txt.gz
- Testing Compressed Files
gzip -t file.txt.gz
gzip
is commonly used to compress individual files and is often used in combination with other tools and utilities. It produces files with the .gz
extension and these compressed files can save disk space and accelerate data transfer when distributing or storing files.
Lynx
lynx
is a text-based web browser that allows users to browse the internet and view web pages from the command line in Unix-like operating systems. It's a versatile tool for accessing information from websites without requiring a graphical user interface.
While it doesn’t render images or complex layouts like modern graphical browsers, it’s useful for quick access to text-based content, checking links, and accessing web resources in a terminal environment.
Basic Usage
lynx [options] [URL]
Lynx Samples
- Viewing a Web Page
lynx https://www.example.com
- Viewing Page Source
lynx -source https://www.example.com
- Viewing Plain Text Version
lynx -dump https://www.example.com
Rsync
Using the rsync
command in Bash
, you can synchronize the contents of a directory between two locations, either locally or across different machines over a network.
Basic Usage
rsync [options] source_directory destination
Rsync Samples
- Local Sync
rsync -av source/ destination/
- Remote Sync over SSH
rsync -av source/ user@remote_host:destination/
- Excluding Files with Progress Indicators
rsync -av --progress --exclude '*.txt' source/ destination/
These examples cover some of the common use cases for rsync
. The command offers many more options and functionalities for more complex synchronization scenarios.
Wget
wget
is a command-line utility used for downloading files from the internet. It supports various protocols, including HTTP, HTTPS, FTP, and FTPS. wget
is commonly used to retrieve files and web content from the command line in Unix-like operating systems.
Basic Syntax
wget [options] [URL]
Wget Samples
- Downloading with Custom Output Name
wget -O output.txt https://www.example.com/file.txt
- Recursively Downloading a Website
wget -r https://www.example.com
wget
is a reliable tool for downloading files and web content from the command line. Its simplicity and range of features make it a preferred choice for tasks that involve fetching resources over the Internet.
Thanks for reading! For more resourses and deployment assistance feel free to access:
- 👉 Read more about Bash & related tools
- 👉 Deploy Projects using your own cloud provider (AWS, GCP, Azure)