Behavior Driven Development (BDD)
An in-depth look at this important software development methodology called BDD
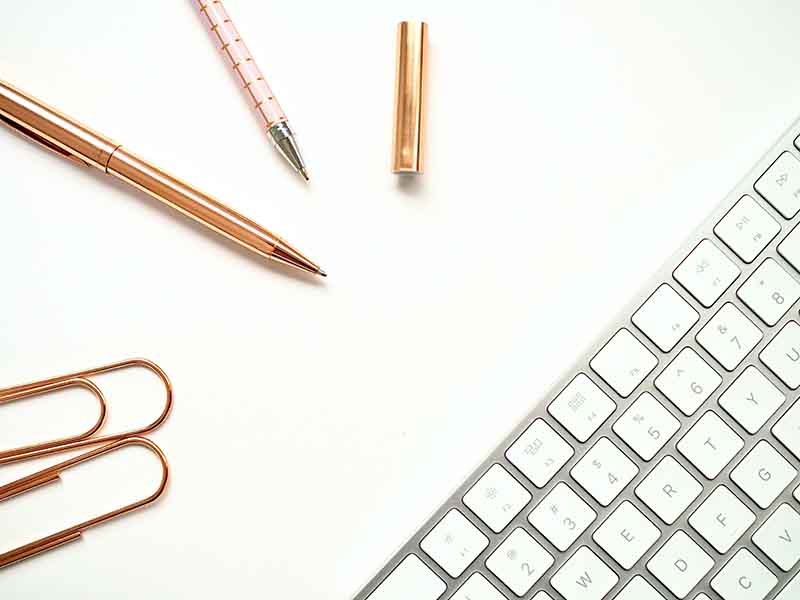
Behavior Driven Development, commonly known as BDD, is an agile software development methodology that aims to bridge the gap between business stakeholders and technical teams. By focusing on the behavior of the system from the user's perspective, BDD helps ensure that software development aligns closely with business goals and user needs. Behavior Driven Development (BDD) is an agile software development approach that encourages collaboration between developers, QA testers, and non-technical or business participants in a software project.
Origins and Philosophy of BDD
BDD was developed by Dan North in the mid-2000s as an evolution of Test Driven Development (TDD). While TDD focuses on testing the implementation of code, BDD shifts the focus to testing the actual behavior of the system as experienced by the user.
The core philosophy of BDD is to describe the behavior of software in a way that both non-technical stakeholders and developers can understand. This shared language helps to reduce misunderstandings and ensures that everyone involved in the project has a clear, common vision of what needs to be built. BDD typically involves three main components
Ubiquitous Language
This is a common vocabulary used by both business stakeholders and developers to describe the system's behavior. It helps ensure that everyone is on the same page and reduces misunderstandings.
User Stories
These are short, simple descriptions of a feature told from the perspective of the person who desires the new capability, usually a user or customer of the system.
Acceptance Criteria
These are conditions that a software product must satisfy to be accepted by a user, customer, or other stakeholder. In BDD, these are often written in a specific format called Gherkin.
The BDD Process
The Behavior Driven Development process typically follows these steps:
- Define User Stories: The team collaborates to write user stories that describe the desired features from the user's perspective.
- Write Scenarios: For each user story, the team writes scenarios in the Gherkin syntax, which follows a Given-When-Then format. For example: Given I am on the login page When I enter valid credentials Then I should be redirected to the dashboard
- Implement Features: Developers write code to implement the features described in the scenarios.
- Automate Tests: The scenarios are translated into automated tests, often using tools like Cucumber, SpecFlow, or JBehave.
- Run Tests: The automated tests are run to verify that the implemented features behave as expected.
- Refine and Repeat: Based on the test results and feedback, the team refines the implementation and repeats the process.
How BDD works
To demonstrate how BDD works in practice, let's walk through the process of developing a new presentation website with key features including a homepage, user authentication, and a contact page.
Setting the Stage: Our Presentation Website Project
Imagine we're tasked with creating a new website for a company to showcase its products and services. The stakeholders have outlined the following high-level requirements:
- A visually appealing homepage that introduces the company
- User authentication system (sign up, log in, log out)
- A contact page for visitors to send inquiries
Let's apply the BDD process to this project, starting with defining user stories and then creating scenarios for each story. Here are the user stories and scenarios:
Homepage Feature Scenario
User Story: As a visitor, I want to see an informative homepage so that I can quickly understand what the company offers.
Scenario: Visitor views the homepage
- Given I am a new visitor to the website
- When I navigate to the homepage
- Then I should see the company logo
- And I should see a headline describing the company's main offering
- And I should see a brief company description
- And I should see navigation links to other pages including "Log In" and "Contact Us"
User Authentication Feature Scenario
User Story: As a new user, I want to create an account so that I can access personalized features.
Scenario: New user signs up successfully
- Given I am on the signup page
- When I enter a valid username, email, and password
- And I click the "Sign Up" button
- Then I should see a confirmation message
- And I should be logged in automatically
Scenario: New user attempts to sign up with existing email
- Given I am on the signup page
- When I enter a username and password
- And I enter an email that is already registered
- And I click the "Sign Up" button
- Then I should see an error message stating "Email already in use"
- And I should remain on the signup page
User Story 2: As a registered user, I want to log in to my account so that I can access my personalized dashboard.
Scenario: Registered user logs in successfully
- Given I am on the login page
- When I enter my correct email and password
- And I click the "Log In" button
- Then I should be redirected to my personalized dashboard
Scenario: User attempts to log in with incorrect credentials
- Given I am on the login page
- When I enter an incorrect email or password
- And I click the "Log In" button
- Then I should see an error message stating "Invalid email or password"
- And I should remain on the login page
User Story 3: As a logged-in user, I want to log out so that I can secure my account when using a shared computer.
Scenario: User logs out
- Given I am logged in to my account
- When I click the "Log Out" button in the navigation menu
- Then I should be logged out
- And I should be redirected to the homepage
- And I should see a "Log In" option in the navigation menu
Contact Page Feature
User Story: As a visitor, I want to send a message to the company so that I can inquire about their products or services.
Scenario: Visitor submits a valid contact form
- Given I am on the contact page
- When I fill in my name, email, and message
- And I click the "Send Message" button
- Then I should see a confirmation message "Your message has been sent"
- And the form should be cleared
Scenario: Visitor attempts to submit an incomplete contact form
- Given I am on the contact page
- When I leave the name or email or message field empty
- And I click the "Send Message" button
- Then I should see an error message indicating the required fields
- And the form should not be submitted
Implementing BDD in the Development Process
With these user stories and scenarios defined, the development team can now proceed with implementation following the BDD approach
Write Automated Tests
Using a BDD framework like Cucumber, SpecFlow, or JBehave, the team would translate these scenarios into automated tests. For example, using Cucumber with Ruby:
Feature: Homepage
Scenario: Visitor views the homepage
Given I am a new visitor to the website
When I navigate to the homepage
Then I should see the company logo
And I should see a headline describing the company's main offering
And I should see a brief company description
And I should see navigation links to other pages including "Log In" and "Contact Us"
Develop Features
Developers would then write the code to implement these features, ensuring that each scenario passes its corresponding automated test.
Continuous Integration
As features are developed, the automated tests would be run as part of a continuous integration process to ensure that new changes don't break existing functionality.
Iterative Refinement
Based on stakeholder feedback and new requirements, the team would continue to add new scenarios or modify existing ones, updating the implementation accordingly.
Benefits of BDD in This Project
By using BDD for our presentation website project, we gain several advantages
Clear Communication
The user stories and scenarios provide a clear, non-technical description of the website's required functionality, ensuring all team members and stakeholders have a shared understanding.
Focus on User Experience
By framing features from the user's perspective, we ensure that the development process remains focused on delivering value to the end-user.
Living Documentation
The scenarios serve as both specification and test cases, providing up-to-date documentation of how the website should behave.
Early Bug Detection
By writing scenarios before implementation, potential issues or inconsistencies in requirements can be identified and addressed early in the development process.
Confidence in Changes
As the project evolves, the comprehensive suite of automated tests gives the team confidence to make changes without fear of unintentionally breaking existing features.
Conclusions on BDD Methodology
This example demonstrates how Behavior Driven Development can be applied to a real-world web development project. By using BDD, we ensure that our presentation website not only meets the technical requirements but also delivers the expected user experience. The collaborative nature of BDD helps align the development team with business goals, resulting in a final product that truly meets user needs and expectations.
As with any methodology, the success of BDD depends on consistent application and buy-in from all team members. When implemented effectively, it can lead to higher quality software, improved team communication, and a development process that remains closely aligned with business objectives throughout the project lifecycle.
Thank you for reading! To get in touch with us, feel free to access:
- 👉 Support Page - chat with the team behind AppSeed
- 👉 Custom Development Services section and get a quote