Django Forms
Learn how to style Django forms using plain Bootstrap and Crispy Forms, a popular third-party library.
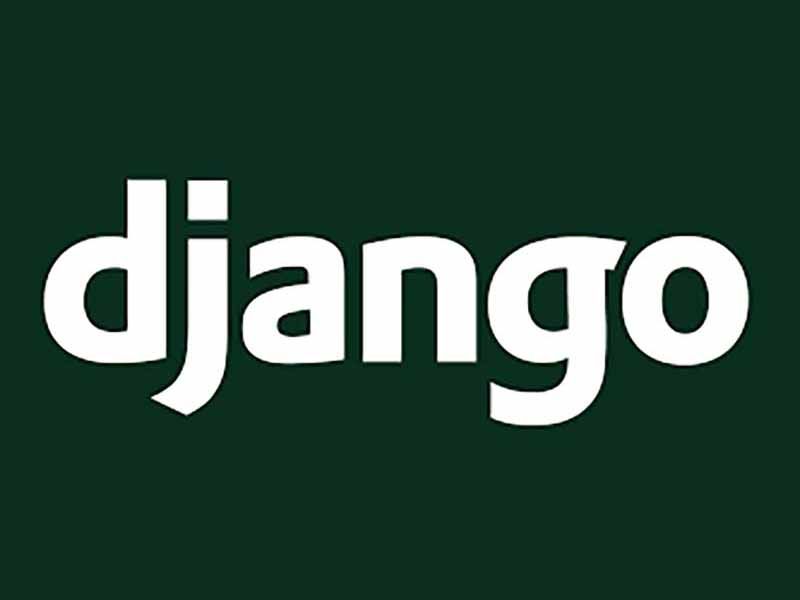
Hello Coders! This article explains how to style forms in Django, a common task in many projects. Styling Django forms with Bootstrap is a popular choice because it allows you to create attractive and responsive forms easily.
Here are the steps to style Django forms with Bootstrap:
✅ Include Bootstrap CSS and JavaScript
First, make sure you have included Bootstrap CSS and JavaScript in your project. You can do this by linking to Bootstrap's CSS and JavaScript files in your HTML template. You can either download Bootstrap and host it locally or use a content delivery network (CDN).
In your HTML template, include the following lines inside the <head>
section:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<script src="https://code.jquery.com/jquery-3.5.1.slim.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.0/dist/js/bootstrap.bundle.min.js"></script>
Make sure to update the URLs to the latest version of Bootstrap if needed.
✅ Use Bootstrap CSS Classes
In your Django template, use Bootstrap's CSS classes to style your form elements. For example, you can apply Bootstrap classes to form fields, buttons, and other elements.
Here's an example of styling a Django form using Bootstrap classes:
<form method="post" class="form">
{% csrf_token %}
<div class="form-group">
{{ form.username.label_tag }}
{{ form.username|add_class:"form-control" }}
</div>
<div class="form-group">
{{ form.password.label_tag }}
{{ form.password|add_class:"form-control" }}
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
In the example above, we're using Bootstrap classes like form
, form-group
, and form-control
to style the form and its elements. You can customize the styling further by adding additional Bootstrap classes as needed.
✅ Styling Form Errors
To style form errors using Bootstrap, you can check if a field has an error in your template and add the is-invalid
class to the form field, or text-danger
for the error message.
Here is a sample:
<form method="post" class="form">
{% csrf_token %}
<div class="form-group">
{{ form.username.label_tag }}
{{ form.username|add_class:"form-control" }}
{% if form.username.errors %}
<small class="text-danger">{{ form.username.errors }}</small>
{{ form.username|add_class:"is-invalid" }}
{% endif %}
</div>
<!-- Rest of the form -->
</form>
✅ Customizing Styles
You can also customize the styling by creating your CSS to override Bootstrap styles or create your own custom classes. This allows you to achieve a unique look for your forms while still utilizing Bootstrap's responsive design.
By following these steps, you can style your Django forms with Bootstrap to create visually appealing and responsive forms that are easy to work with.
✅ Django Crispy Forms
Another choice is to use Django Crispy Forms, a popular third-party library that simplifies the rendering of Django forms by making them easy to style and customize using the Bootstrap framework (and other front-end frameworks).
It provides a template pack that allows you to render forms in a more visually appealing and efficient manner, especially when working with Bootstrap.
Below are the steps to integrate Crispy Forms with Django:
Installation
First, install the django-crispy-forms
package using pip
:
pip install django-crispy-forms
👉 Configuration
Add 'crispy_forms'
to your INSTALLED_APPS
in your Django project's settings file (settings.py
):
INSTALLED_APPS = [
# ...
'crispy_forms',
]
👉 Specify the Crispy Form Template Pack
In your settings.py
, set CRISPY_TEMPLATE_PACK
to 'bootstrap4'
or 'bootstrap5'
depending on your Bootstrap version:
CRISPY_TEMPLATE_PACK = 'bootstrap4'
👉 Using Crispy Forms in Templates
To use Crispy Forms in your Django templates, load the crispy_forms_tags
template tag at the top of your template, and apply the crispy
filter to your form:
{% load crispy_forms_tags %}
<form method="post" class="form">
{% csrf_token %}
{{ form|crispy }}
<button type="submit" class="btn btn-primary">Submit</button>
</form>
The |crispy
filter renders the form fields in a more structured and Bootstrap-friendly way.
👉 Customizing Form Layout
You can further customize the layout and styling of your forms using various template packs provided by Crispy Forms. For example, you can create custom layouts, modify field orders, and apply custom CSS classes.
To customize the form layout, you can create a layout using the Layout
class from Crispy Forms and apply it to your form.
from crispy_forms.helper import FormHelper
from crispy_forms.layout import Layout, Field
class MyForm(forms.Form):
# Your form fields
helper = FormHelper()
helper.form_method = 'post'
helper.layout = Layout(
Field('field1', css_class='my-custom-class'),
Field('field2', css_class='another-custom-class'),
# Add more fields and customizations here
)
👉 Advanced Features
Django Crispy Forms offers many advanced features, including support for different front-end frameworks, integration with form sets, form error handling, custom template packs, and more. You can explore these features in the official documentation.
Django Crispy Forms simplifies the process of rendering and styling forms in your Django project, especially when working with Bootstrap. It's a valuable tool for creating visually appealing and user-friendly forms in your web applications.
✅ Resources
- 👉 Access AppSeed for more starters and support
- 👉 Deploy Projects on Aws, Azure, and DO via DeployPRO
- 👉 Build apps with Django App Generator (free service)