Django - Introduction for Beginners
This article is a short introduction to Django, the most popular Python web Framework.
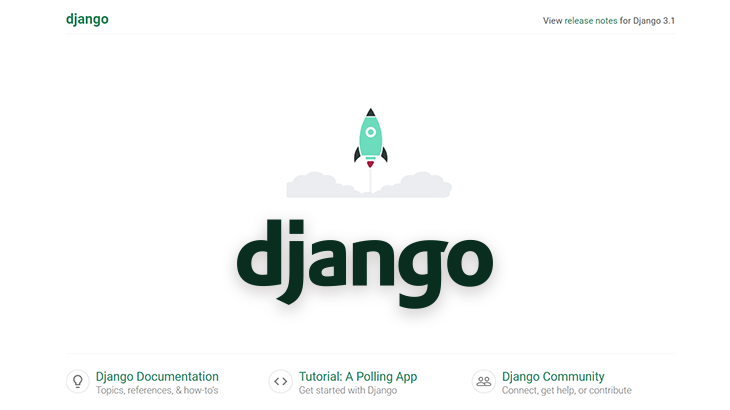
This article is a short introduction to Django, the most popular Python web Framework. For newcomers, Django was initially developed in 2003 and since than has become a reference framework in the web development ecosystem mostly for "batteries-included" concept and built-in security patterns coded by experienced developers. At the moment this article is published, Django scores 55k Github Starts, 24k Forks and has an active community with 2k+ contributors.
Thanks for reading! Topics covered and resources:
- Django - why using it
- Scaffolding a Django Project using command line
- Django Configuration
- Django Template Language
- Django Admin Section
- Django Static Files
- Django production checklist
- Django Samples - Production-ready Starters
Django - Reasons to use it
With a long history behind it and actively supported by many open-source enthusiasts, Django comes with the “batteries included” philosophy and provides almost everything developers might want to do “out of the box”. Being in production for more than 15 years, the Django community members released in the open-source ecosystem many useful libraries. I will mention just a few below:
- Django Extensions - provides helpers like shell_plus, clean_pyc
- Django-environ - manage the environment variables with ease
- Django-click - helps you write Django management commands
- Django REST framework - a flexible toolkit for building Web APIs
- Django REST Swagger - Swagger/OpenAPI Doc Generator for Django
- Django-Allauth - Unlocks 3rd party (social) authentication: Google, Github
- Django Model Utils - model mixins and utilities for Django
- Django Contact Form - helps developers manage forms with ease
- Django import/export - manage data with included admin integration.
On top of this, Django has other strong points that improve the scalability, security, and long-term maintenance of a project.
Versatile – Django can be used to code from simple, presentation websites to blog platforms or heavy traffic, eCommerce platforms. many big companies like Instagram or Disqus choose Django as their core backend technology.
Secure – Django comes with a built-in security pattern coded by experienced developers. Django helps developers avoid many common security mistakes by providing modules and layers that have been engineered and tested to protect the website automatically.
Complete – Django follows the “Batteries included” philosophy and provides almost everything developers might want to do “out of the box”. Once the developers learn the framework, they can do almost anything from simple websites. API’s, microservices, or complex eCommerce platforms.
To start coding a simple Django is super easy. Let's scaffold a new, simple project using the command line.
Scaffolding a Django Project
The goal of this section is to help beginners to generate a Django project using the console. Before we start, we must check if Python is properly installed and accessible via the terminal. The full setup to generate a simple project is presented below:
1# - Check Python version
$ python --version
Python 3.8.4
2# - Create and active a virtual environment
$ # Virtualenv modules installation (Unix based systems)
$ virtualenv env
$ source env/bin/activate
Using a Virtual is optional but recommended - to read more about virtual environments, please access this tutorial - Python Virtual Environments.
3# - Install Django - using PIP, the package manager for Python
$ pip instal django
4# - Create our first Django project
$ django-admin startproject firstdjango
This command will create a new project/directory "firstdjango" with this minimal structure:
firstdjango/
manage.py
firstdjango/
__init__.py
settings.py
urls.py
asgi.py
wsgi.py
5# - Start the project
$ cd firstdjango
$ python manage.py runserver
...
Django version 3.1.7, using settings 'firstdjango.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
At this point, we can visit our first Django project in the browser and see a simple page with a layout similar to this screenshot:
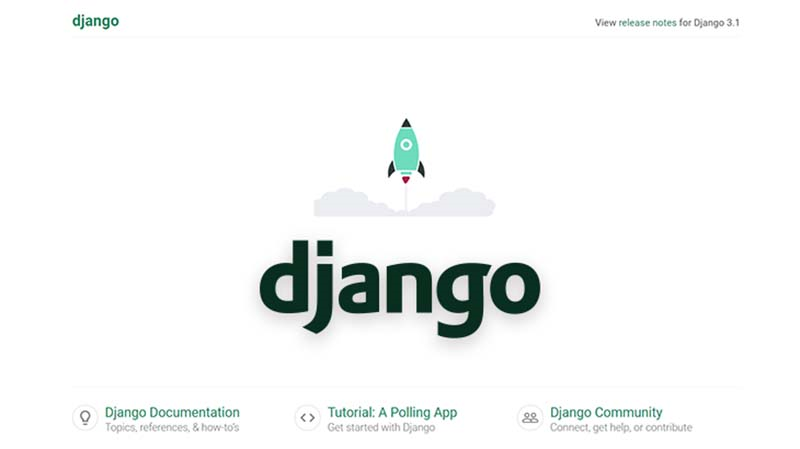
Being a generated project, it might be a good idea to take a look at the project structure and analyze the configuration.
Django Configuration
The Django settings file is specified by "manage.py" - the bootstrap scripts located in the project root.
# manage.py contents
import os
import sys
def main():
os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'firstdjango.settings')
...
if __name__ == '__main__':
main()
The "settings.py" file contains many settings that can be used to customize Django. The most important ones are:
- SECRET_KEY - used for cryptographic operations for passwords, tokens, etc.
- DEBUG - useful in development to get more information regarding errors
- ROOT_URLCONF - the routing file used by Django to resolve requests
- TEMPLATES - the directory where HTML templates are saved
- DATABASES - settings to connect to the database
Django supports all popular databases: PostgreSQL, MariaDB, MySQL, Oracle and SQLite, the default one. If we open the settings file and search the DATABASES node, we should see that SQLite is used by default:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': BASE_DIR / 'db.sqlite3',
}
}
To read more about Django configuration options, please access:
- Django Settings - the official help
- Configuring Django - Best Practices Guide
Django Template Language
Django provides a powerful template language used to manage application HTML files with ease. Components common to many pages (footer, header) can be defined separately and reused in different places using dynamic information. An example might be the top menu that shows "LOGIN" option for guests users and "LOGOUT" for authenticated ones. Let's take a look and explore a few basic features.
Template Variables -
{{ username }}
When the template is processed and a variable is detected, Django will search the value in the current context. For instance, if the username has the value "John" and the template is something like this:
<p>
Your username is {{ username }}
</p>
The generated HTML file will be:
<p>
Your username is John
</p>
Filters
- {{ name | lower }} - convert variable to lowercase
- {{ info | truncatewords:5 }} - display the first 5 words of the info variable
- {{ value | default:"NULL" }} - shows a default value when "value" is empty
Tags
Tags are using a different syntax "{% tag %}" and are used in conditional output and loops.
For Tag - can be used to iterate over lists:
<ul>
{% for user in all_users %}
<li>{{ user }}</li>
{% endfor %}
</ul>
If, else Tags - Test is a variable exists
{% if all_users %}
Registered Users: {{ all_users|length }}
{% else %}
No Users Found.
{% endif %}
To read more about Django template engine please access:
- Django template language - the official help
- Introduction to Django Templates - a comprehensive tutorial
Once we have a basic understanding of the Django Template System, we can move our attention to the next topic - Django Admin Section.
Django Admin Section
Django comes with a powerful administration interface that is actually a standalone Django app with a simple and intuitive interface. To access the administration module, we need to create a special user, a "superuser" using the Django console.
$ python manage.py createsuperuser
$ Username (leave blank to use 'test'): admin
$ Email address: admin@appseed.us
$ Password: ********
$ Password (again): ********
Superuser created successfully.
The above code creates a "superuser" admin and now we can access the administration module and visualize the options.

Admin section allows us to add/remove/update users and interact with all tables defined in the database. Each user can be edited to get access to specific operations (view for instance) and disallow others (create or update).
To read more about Django Admin interface please access:
- Django admin site - the official documentation
- Django admin site - Mozilla Web Docs
Django Static Files
Web apps are provided, most of the time, along with resources used to style and improve the UI: CSS files, JS, and images. Django assists us to manage the assets with ease via a built-in app called "staticfiles
" that exposes a few helpers specialized for static files management:
- "static" template tag - used as a short URL for any asset used by HTML files
- "static" view - that works in ONLY in development mode
- "collectstatic" - a useful command that isolates all assets in a separate directory
Django configuration regarding static files
- DEBUG - this flag should be set to True (development mode)
- INSTALLED_APPS should have 'django.contrib.staticfiles' (present by default)
- STATIC_URL - with a default value '/static/'
To use the "static" tag in an HTML template file is quite easy:
{% load static %}
<img src="{% static 'assets/photo.jpg' %}" alt="Cool image">
On disk, the file has a physical path as shown below:
<PROJECT ROOT>
manage.py # Load settings from core.settings
core/settings.py # Core settings
core/static # Assets root directory
core/static/assets/photo.jpg # The used asset
We can also use a hardcoded path, without "static" tag:
<img src="/static/assets/photo.jpg" alt="Cool image">
DEBUG = False
This is a super important aspect that affects the deployment of the project in production and we can solve it using "collectstatic" command:
$ python manage.py collectstatic
This command collects all static files from all apps folders (including the static files for the admin app) and copies them into STATIC_ROOT directory defined in the "settings.py" file.
Once we have all files in one place, the project is ready to be deployed in production.
Django Production Checklist
Production means to release and install our product to be used by real users on a real public domain (www.yoursite.com) or as a private service in a private (intranet) network.
The deployment checklist provided in the official Django might help beginners to understand better the process and required changes in order to have a successful deployment - The short-list with required changes are listed below
SECRET_KEY - Configuration Parameter
The value should be kept secret and is a good idea to use a long (25 chars or more), random string. The value can be provided in the Settings file in multiple ways:
- Hardcoded value in "settings.py" file
- Load the value from the environment
- Read the value from a file
DEBUG - Configuration Parameter
By setting DEBUG to false, a few things are automatically activated in Django core
- ALLOWED_HOSTS variable must be set to match the IP or Public Domain
- Static files - are no longer managed by Django
- When runtime errors occur, the debug information is replaced with the default 500 error page
ALLOWED_HOSTS - Configuration Parameter
This setting contains a list of strings representing the host/domain names that this Django site can serve. Accepted values are fully domain names like "www.appseed.us" or subdomain wildcards ".example.com" to accept the deployment on any subdomain of "example.com".
Valid Samples (case insensitive, not including port)
- ALLOWED_HOSTS = ['appseed.us', 'www.appseed.us']
- ALLOWED_HOSTS = ['.appseed.us'] - subdomain wildcard
When DEBUG is True, and ALLOWED_HOSTS is empty, the default values are " ['.localhost', '127.0.0.1', '[::1]']" - any localhost subdomain and the localhost IP Address.
CSRF_COOKIE_SECURE - Configuration Parameter
Must be set to True to avoid transmitting the CSRF cookie over HTTP accidentally.
Once we have set all the above settings we can run an extra check by executing the command "check deploy"
$ manage.py check --deploy
Based on the returned output we can iterate until all checks are ok.
To move forward from theory to practice, I will mention a few projects already coded using Django best-practices patterns on top of modern UI Kits. All are free, released under permissive licenses on Github (no account required to use the sources).
Datta Able Django
Datta Able Bootstrap Lite is the most stylized Bootstrap 4 Lite Admin Template, around all other Lite/Free admin templates in the market. It comes with high feature-rich pages and components with fully developer-centric code. Before developing Datta Able our key points were performance and design.
- Datta Able Django - the product page
- Datta Able Django Demo - LIVE Deployment
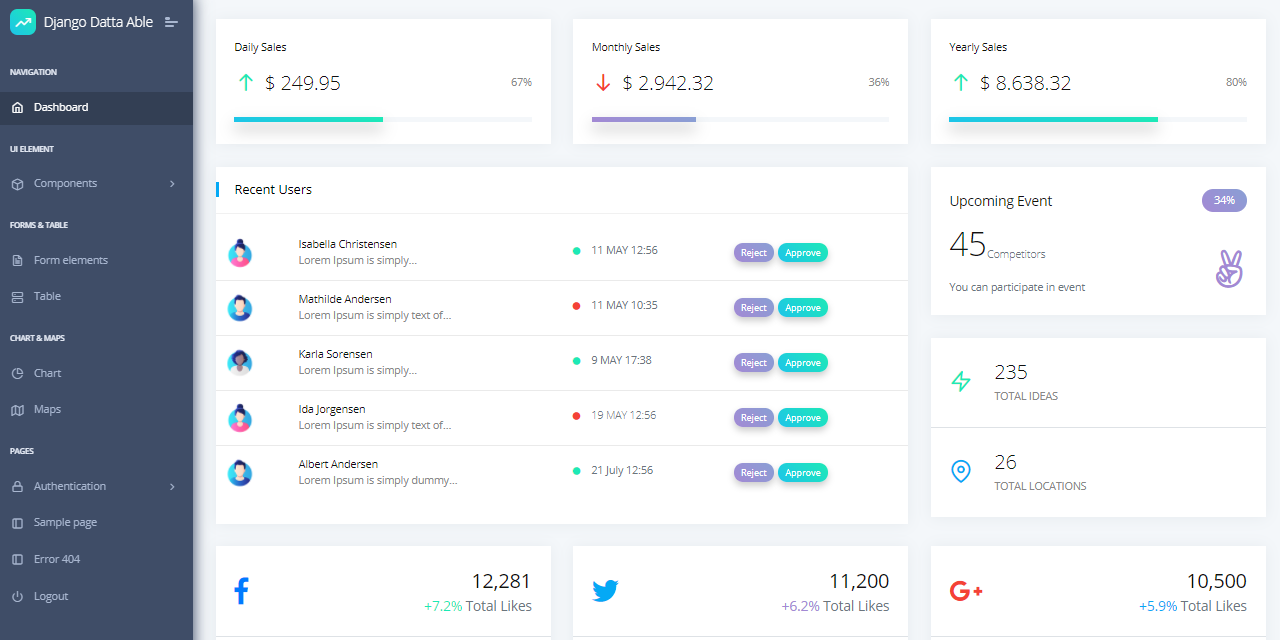
Django Dashboard Argon
Argon Dashboard is built with over 100 individual components, giving you the freedom of choosing and combining. All components can take variations in color, that you can easily modify using SASS files. You will save a lot of time going from prototyping to full-functional code, because all elements are implemented.
- Django Dashboard Argon - Product page
- Django Dashboard Argon Demo - LIVE Deployment

Django Bootstrap 5 Volt
Volt Dashboard is a free and open source Bootstrap 5 Admin Dashboard featuring over 100 components, 11 example pages and 3 plugins with Vanilla JS. There are more than 100 free Bootstrap 5 components included some of them being buttons, alerts, modals, datepickers and so on.
- Django Bootstrap 5 Volt - product page
- Django Bootstrap 5 Volt - Demo - LIVE deployment
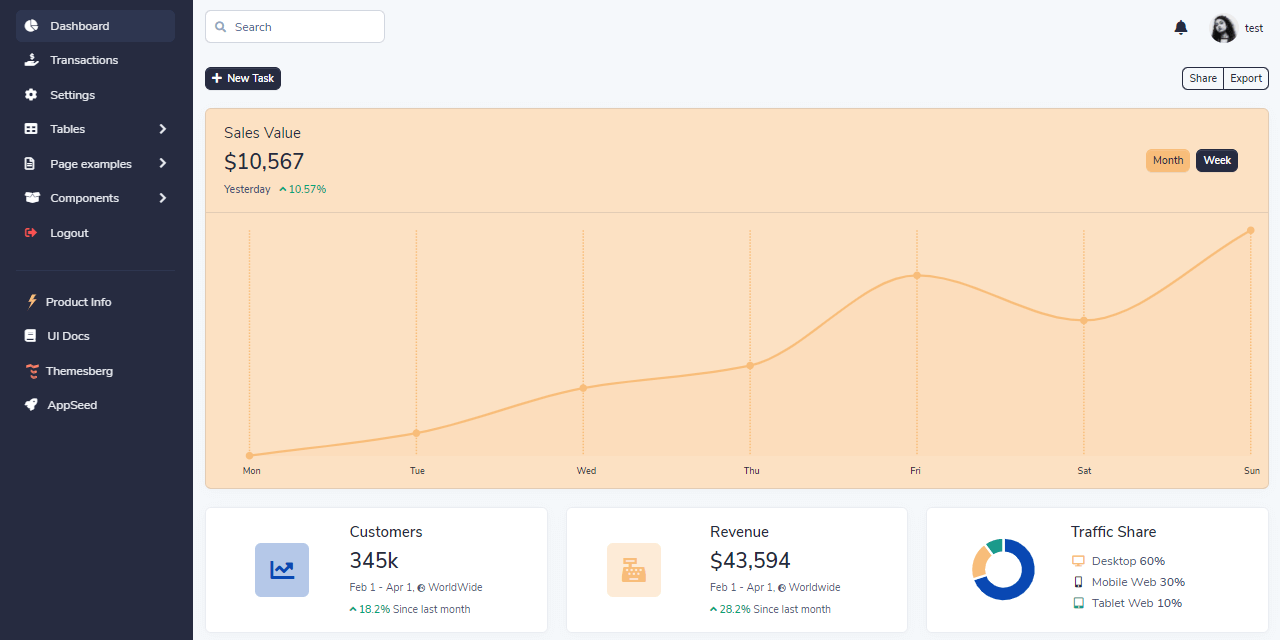
Django Dashboard Material
Material Dashboard is a free Material Bootstrap 4 Admin with a fresh, new design inspired by Google's Material Design. Material Dashboard comes with 5 color filter choices for both the sidebar and the card headers (blue, green, orange, red and purple) and an option to have a background image on the sidebar.
- Django Dashboard Material - product page
- Django Dashboard Material - Demo - LIVE App
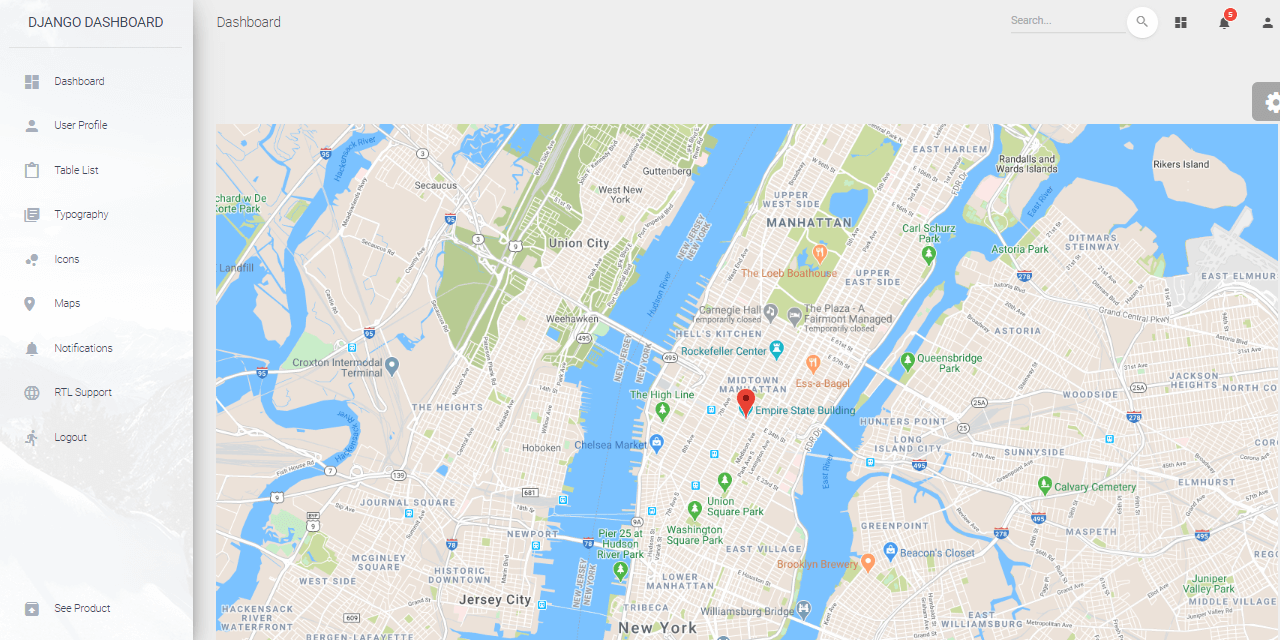
Django Atlantis Dark
Atlantis Lite (Dark Design) is a free bootstrap 4 admin dashboard that is beautifully and elegantly designed to display various metrics, numbers or data visualization. Atlantis Lite admin dashboard has 2 layouts, many plugins and UI components to help developers create dashboards quickly and effectively so they can save development time and also help users to make the right and fast decisions based on existing data.
- Django Atlantis Dark - Product page
- Django Atlantis Dark - Demo - LIVE Deployment
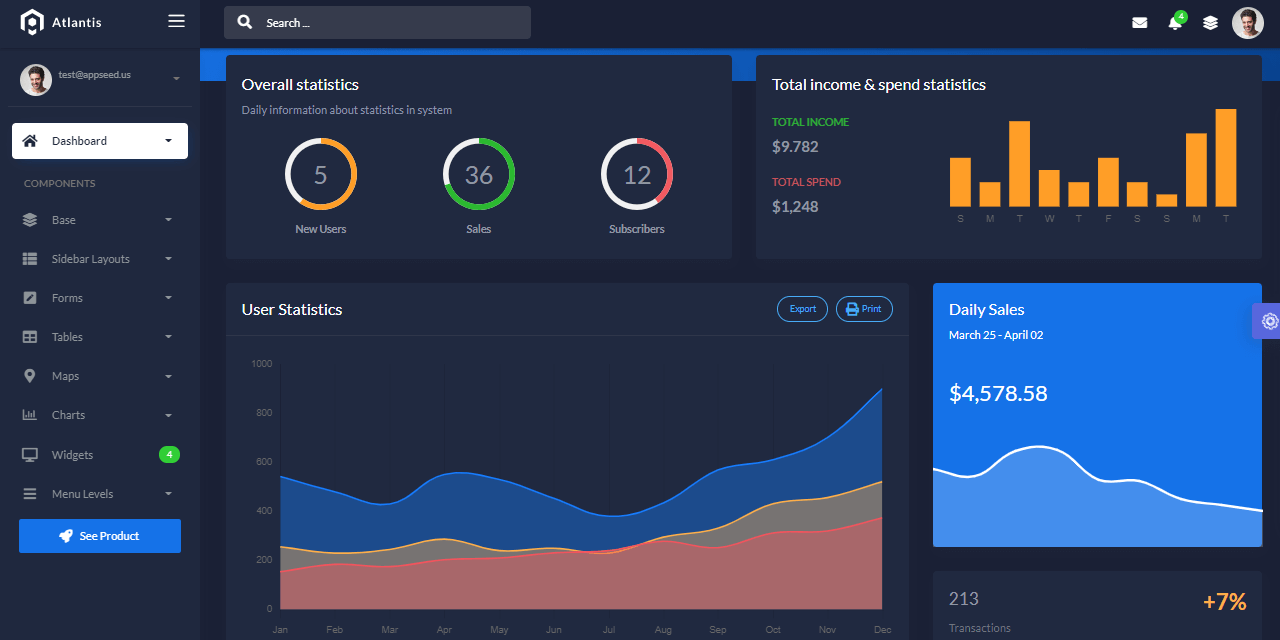
Star Admin Django
Star Admin is a beautifully designed admin template featuring a fine selection of useful Bootstrap components and elements. The pre-built pages of the templates are intuitive and very well-designed. The pre-built pages of the templates are intuitive and very well-designed. Star Admin is sure to make your development process truly enjoyable.
- Star Admin Django - product page
- Star Admin Django Demo - LIVE Deployment
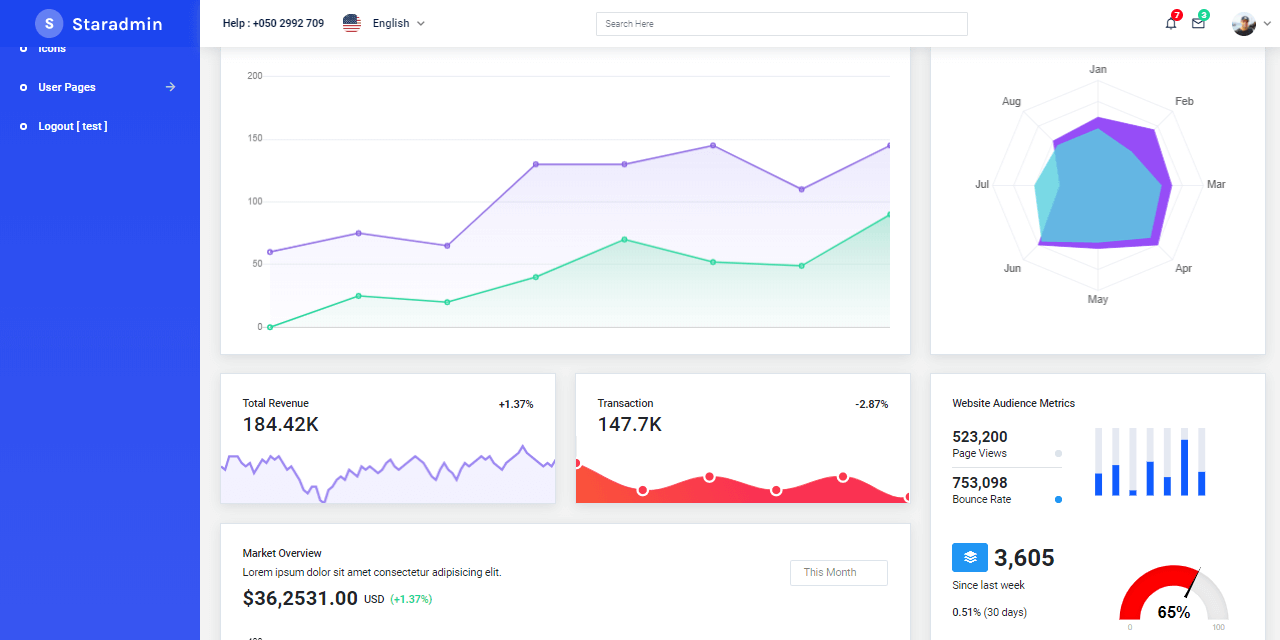