Dynamic API for Django - Free PyPi Library
An open-source PyPi Library that builds automatically Dynamic APIs on top of DRF with minimum effort - Released under the MIT License on Github.
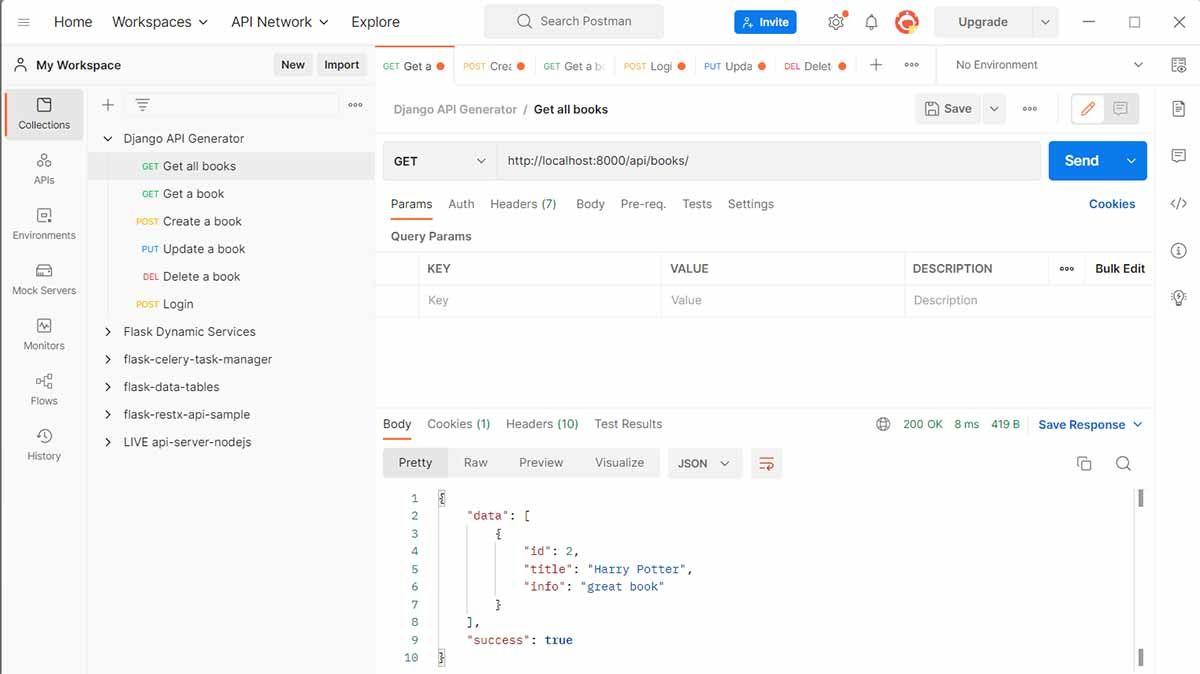
Hello! This article presents an open-source library that builds automatically API services for any model defined in a Django application. Once the Dynamic API library is installed, any model enabled in the configuration becomes manageable via a DRF interface secured by JWT tokens. The sources, published on GitHub under the MIT License, can be used in commercial projects or eLearning activities. Thanks for reading!
- π Dynamic API for Django - source code
- π Dynamic API for Django - PyPi page
This library is basically an abstract layer that connects any app model with DRF without writing any code. The coding pattern uses dynamic routing (no model name specialization) and the dynamic imports of the model definition at runtime.
A quick overview of this tool can be found on YouTube - Dynamic API
β¨ Library Core Features
The product can be integrated with any Django project using PIP:
$ pip install django-dynamic-api
Once the library is installed, we should be able to activate API services on top of any app model in no time.
- β
API engine
provided byDRF
- β
Secured by
JWT Tokens
(mutating requests) - β
Minimal Configuration
(single line in the config for each model) - β GET requests are public
- β Update, Delete, and Creation requests are protected
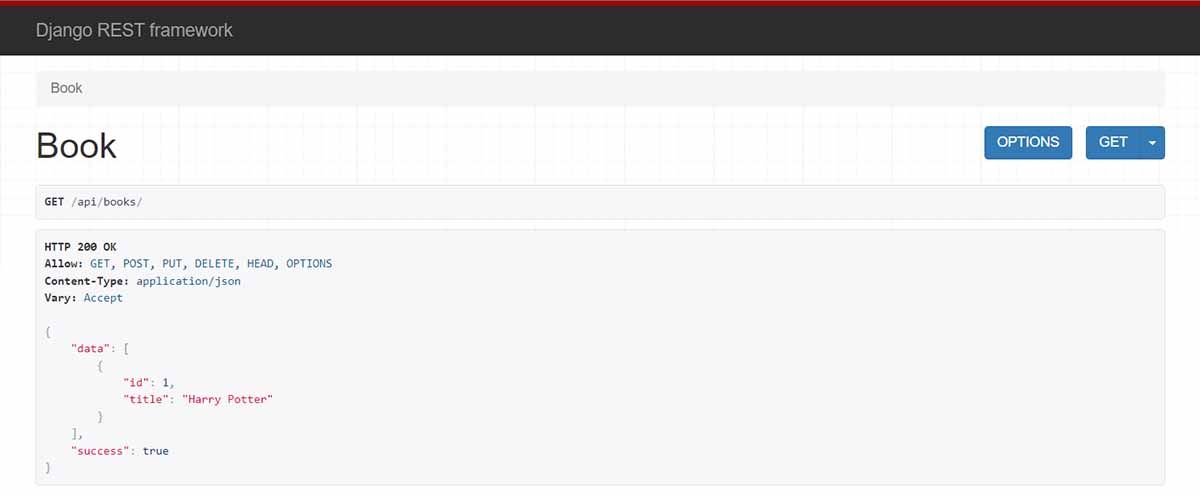
β¨ How to use the product
This section presents all the necessary steps to code a simple Django project with a production-ready API provided by the Dynamic API library.
π Step #1 - Clone a basic Django Starter: Core Django
This simple codebase comes with minimal structure and Docker support. Feel free to use your own codebase.
$ git clone https://github.com/app-generator/core-django.git
$ cd core-django
π Step #2 - Create virtual environment and install Django
$ virtualenv env
$ source env/bin/activate
$ pip install -r requirements.txt
π Step #3 - Migrate database
$ python manage.py makemigrations
$ python manage.py migrate
π Step #4 - Create SuperUser and start the app
$ python manage.py createsuperuser
$ python manage.py runserver
The superuser credentials are required later in API usage.
At this point, we have a basic Django starter ready to be used and extended with more features. Let's move on with our tutorial and code a simple app that defines a Book
model.
π Step #5 - Create a new APP
$ django-admin startapp app1
This command executed in the root of the project will create a skeleton app. In order to make it more useful, we will define a simple model, managed later by the Dynamic API library.
class Book(models.Model):
title = models.CharField(max_length=100)
info = models.CharField(max_length=100, default='')
π Step #6 - Configure the new app and migrate the database
Each time a Django project got a new app, the configuration should be updated in order to actually use it in the code:
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"app1", # <-- NEW
]
The migration step is also mandatory for all the new apps: Β
$ python manage.py makemigrations
$ python manage.py migrate
Our sample Django codebase is stable and has a model ready to be used via the Dynamic API library. Let's install the tool and use it.
π Step #7 - Install Dynamic API Package
$ pip install django-dynamic-api
π Step #8 - Update APPs section to include the library
INSTALLED_APPS = [
...
'django_dyn_api', # Django Dynamic API # <-- NEW
'rest_framework', # Include DRF # <-- NEW
'rest_framework.authtoken', # Include DRF Auth # <-- NEW
...
]
At the end of the settings file, we need to add a new section used by the DYNAMIC Api module:
DYNAMIC_API = {
# API_SLUG -> Import_PATH
'books' : "app1.models.Book",
}
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'rest_framework.authentication.SessionAuthentication',
'rest_framework.authentication.TokenAuthentication',
],
}
DYNAMIC_API
section informs the library to manage the Book model under the books
API route.
This configuration part is crucial in order to have a stable API service.
π Step #9 - Migrate (again) the database
The migration is required by the DRF library, especially by the JWT token management part. Β Β
$ python manage.py makemigrations
$ python manage.py migrate
π Step #10 - Update configuration
from django.contrib import admin
from django.urls import path, include # <-- NEW: 'include` directive
from rest_framework.authtoken.views import obtain_auth_token # <-- NEW
urlpatterns = [
path("admin/", admin.site.urls),
path('', include('django_dyn_api.urls')), # <-- NEW: API routing rules
path('login/jwt/', view=obtain_auth_token), # <-- NEW
]
At this point, the new API can be accessed in the browser via 3rd party tools like POSTMAN.
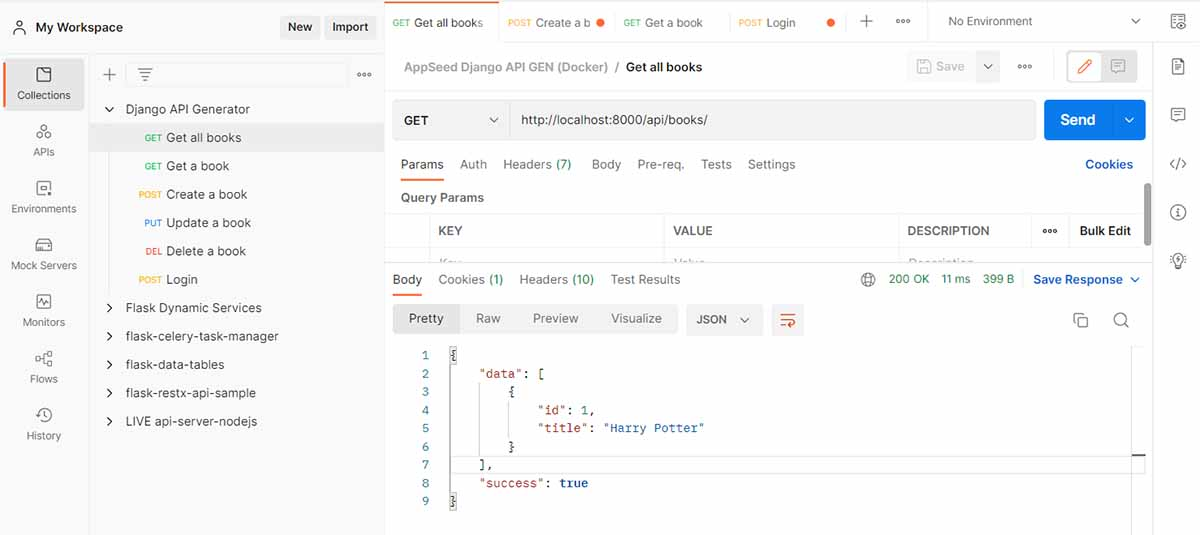
The full source code used in this demonstration can be found on GitHub, available for curious minds as reference. Β
Thanks for reading! For more tools and support feel free to access:
- π More Developer Tools provided by AppSeed
- π Β Free support via Email & Discord (just in case)