How to Generate Source Code
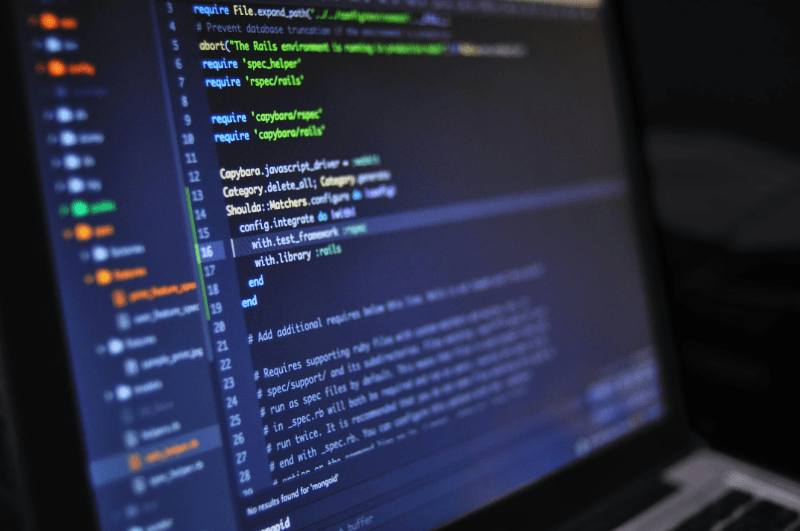
Generating source code has become an increasingly valuable practice in the world of software development. It's a process that involves creating code automatically, rather than writing it manually line by line. This approach can significantly streamline development workflows, reduce errors, and boost productivity.
At its core, code generation is about automating repetitive tasks. Instead of developers writing similar code structures over and over, they can use tools or scripts to produce code based on predefined templates or rules. This not only saves time but also ensures consistency across the codebase.
There are several well-known ways to generate source code. One common method is using template engines, which allow developers to define reusable code structures with placeholders for variable content. When the template is processed, these placeholders are filled with specific data to produce the final code.
Another popular approach is using domain-specific languages (DSLs). These are specialized languages designed for a particular application domain. Developers can write high-level specifications in the DSL, which are then translated into full-fledged code in a general-purpose programming language.
Code generation can also be achieved through metaprogramming techniques. This involves writing code that can modify or generate other code at compile time or runtime. Many modern programming languages, including Python, offer powerful metaprogramming capabilities.
In recent years, AI-powered code generation has gained traction. Tools like GitHub Copilot use machine learning models trained on vast code repositories to suggest or auto-complete code based on natural language prompts or partial code snippets.
Let's look at a practical example of code generation using Python. We'll create a simple script that generates a basic Python class based on user input. This example demonstrates how we can automate the creation of repetitive code structures:
def generate_class(class_name, attributes):
class_template = f"""
class {class_name}:
def __init__(self, {', '.join(attributes)}):
{' '*8}# Initialize attributes
{' '*8}self.{(" = self.\n" + ' '*8).join(attributes)} = {', '.join(attributes)}
def __str__(self):
return f"{class_name}({', '.join([f'{attr}={{self.{attr}}}' for attr in attributes])})"
"""
return class_template
# Example usage
class_name = input("Enter the class name: ")
attributes = input("Enter attributes (comma-separated): ").split(',')
generated_code = generate_class(class_name, attributes)
print("Generated code:")
print(generated_code)
# Optionally, save the generated code to a file
with open(f"{class_name.lower()}.py", "w") as file:
file.write(generated_code)
print(f"Code saved to {class_name.lower()}.py")
This script does the following:
- It defines a
generate_class
function that takes a class name and a list of attributes. - The function creates a string containing a template for a Python class, including an
__init__
method to initialize attributes and a__str__
method for string representation. - The script prompts the user for a class name and attributes.
- It then calls the
generate_class
function with the provided inputs. - The generated code is printed to the console and saved to a file.
When you run this script, it will interactively create a new Python class based on your input. For example, if you enter "Person" as the class name and "name, age, occupation" as attributes, it will generate a Person
class with those attributes, complete with initialization and string representation methods.
This simple example illustrates how code generation can be used to automate repetitive tasks in software development. While this is a basic implementation, the concept can be extended to more complex scenarios, such as generating entire file structures, API clients, or database models based on specifications or schemas.
Domain-Specific Languages
A Domain-Specific Language is a programming language or specification language dedicated to a particular problem domain, a specific problem representation technique, or a defined solution technique. Unlike general-purpose languages like Python or Java, which are designed to solve a wide range of problems, DSLs are tailored to express solutions in a particular domain more effectively.
The key idea behind DSLs is to bridge the gap between the problem domain (what needs to be solved) and the solution domain (how it's solved in code). By providing a language that closely matches the concepts and operations of a specific field, DSLs can make it easier for domain experts to express their intent, even if they're not professional programmers.
There are two main types of DSLs
External DSLs
These are separate languages with their own syntax and parser. Examples include SQL for database queries, regular expressions for pattern matching, or Cucumber for behavior-driven development.
Internal DSLs
These are embedded within a host general-purpose language, leveraging its syntax and execution environment. They're often implemented as fluent interfaces or method chaining in object-oriented languages.
In the context of code generation, DSLs are particularly powerful. They allow developers to express high-level concepts in a concise, domain-specific way, which can then be transformed into more verbose, lower-level code in a general-purpose language.
Here's an expanded example to illustrate how a DSL might be used for code generation in Python. Let's create a simple DSL for defining data models, which we'll then use to generate Python classes:
class Field:
def __init__(self, name, type):
self.name = name
self.type = type
class Model:
def __init__(self, name):
self.name = name
self.fields = []
def add_field(self, name, type):
self.fields.append(Field(name, type))
return self
def generate_model_code(model):
class_code = f"class {model.name}:\n"
class_code += " def __init__(self"
for field in model.fields:
class_code += f", {field.name}: {field.type.__name__}"
class_code += "):\n"
for field in model.fields:
class_code += f" self.{field.name} = {field.name}\n"
return class_code
# Using our DSL to define a model
user_model = (
Model("User")
.add_field("id", int)
.add_field("name", str)
.add_field("email", str)
.add_field("age", int)
)
# Generate Python code from our model
generated_code = generate_model_code(user_model)
print(generated_code)
# Optionally, save the generated code to a file
with open("user_model.py", "w") as file:
file.write(generated_code)
print("Code saved to user_model.py")
In this example:
- We define a simple DSL for creating data models. The
Model
andField
classes form the basis of our DSL. - The
Model
class uses method chaining to provide a fluent interface for adding fields. This is an example of an internal DSL. - We define a
generate_model_code
function that takes our DSL model and generates Python code for a corresponding class. - We use our DSL to define a
User
model with several fields. - Finally, we generate Python code from our model definition and print it.
This DSL allows us to define data models in a concise, readable manner. The code generation step then takes this high-level description and produces the more verbose Python class definition.
The benefits of this approach include:
- Abstraction: The DSL hides the complexity of the full class definition, allowing developers to focus on the essential aspects of the data model.
- Consistency: All generated classes will follow the same structure and conventions.
- Productivity: Defining models is quicker and less error-prone than writing full class definitions by hand.
- Flexibility: Changes to the code generation logic (e.g., adding new features to all models) can be made in one place, affecting all generated code.
This example is relatively simple, but the concept can be extended to more complex scenarios. For instance, you could expand the DSL to include relationships between models, and validation rules, or even generate database schema migrations alongside the Python classes.
DSLs, when combined with code generation, provide a powerful tool for managing complexity in software development. They allow developers to work at a higher level of abstraction, express domain concepts more clearly, and automate the creation of repetitive code structures. As projects grow in size and complexity, these benefits become increasingly valuable, making DSLs a key technique in modern software engineering practices.
Metaprogramming
Metaprogramming is a powerful technique where a program can treat code as data, manipulating or generating code during its own execution. This approach allows for highly dynamic and flexible programming patterns, often leading to more concise and maintainable code.
In Python, metaprogramming is particularly accessible due to the language's dynamic nature and rich set of introspection tools. Some key features that enable metaprogramming in Python include:
- Dynamic attribute access and modification
- Reflection capabilities (type(), isinstance(), etc.)
- Function and class decorators
- Metaclasses
Let's explore a few metaprogramming techniques with examples:
Dynamic Attribute Manipulation
class DynamicAttributes:
def __getattr__(self, name):
return f"Dynamically created attribute: {name}"
def __setattr__(self, name, value):
print(f"Setting {name} = {value}")
super().__setattr__(name, value)
obj = DynamicAttributes()
print(obj.non_existent) # Outputs: Dynamically created attribute: non_existent
obj.new_attr = 42 # Outputs: Setting new_attr = 42
This example demonstrates how we can intercept attribute access and creation, allowing for dynamic behavior.
Function Decorators
Decorators are a common metaprogramming technique in Python. They allow you to modify or enhance functions without changing their source code.
def log_calls(func):
def wrapper(*args, **kwargs):
print(f"Calling {func.__name__} with args: {args}, kwargs: {kwargs}")
result = func(*args, **kwargs)
print(f"{func.__name__} returned {result}")
return result
return wrapper
@log_calls
def add(a, b):
return a + b
add(3, 5) # Outputs:
# Calling add with args: (3, 5), kwargs: {}
# add returned 8
Class Decorators
Similar to function decorators, class decorators can modify or enhance class definitions.
def add_greeting(cls):
def say_hello(self):
return f"Hello, I'm {self.name}"
cls.say_hello = say_hello
return cls
@add_greeting
class Person:
def __init__(self, name):
self.name = name
p = Person("Alice")
print(p.say_hello()) # Outputs: Hello, I'm Alice
Metaclasses
Metaclasses are perhaps the most powerful metaprogramming tool in Python. They allow you to customize class creation itself.
class AutoRepr(type):
def __new__(cls, name, bases, attrs):
def __repr__(self):
return f"{name}({', '.join(f'{k}={v!r}' for k, v in self.__dict__.items())})"
attrs['__repr__'] = __repr__
return super().__new__(cls, name, bases, attrs)
class Person(metaclass=AutoRepr):
def __init__(self, name, age):
self.name = name
self.age = age
p = Person("Bob", 30)
print(p) # Outputs: Person(name='Bob', age=30)
This metaclass automatically adds a __repr__
method to any class that uses it, providing a string representation of the object's attributes.
Code Generation withexec
andeval
:
While powerful, these functions should be used cautiously due to potential security risks if used with untrusted input.
def create_power_function(power):
func_code = f"""
def power_{power}(x):
return x ** {power}
"""
exec(func_code, globals())
create_power_function(3)
print(power_3(4)) # Outputs: 64
This example dynamically creates a new function that raises a number to a specified power.
Abstract Syntax Trees (AST)
For more complex code generation or analysis, you can use Python's ast
module to work with the abstract syntax tree of Python code.
import ast
def add_logging_to_functions(code):
tree = ast.parse(code)
for node in ast.walk(tree):
if isinstance(node, ast.FunctionDef):
log_stmt = ast.Expr(
value=ast.Call(
func=ast.Name(id='print', ctx=ast.Load()),
args=[ast.Str(s=f"Calling function {node.name}")],
keywords=[]
)
)
node.body.insert(0, log_stmt)
return ast.unparse(tree)
original_code = """
def greet(name):
return f"Hello, {name}!"
def farewell(name):
return f"Goodbye, {name}!"
"""
modified_code = add_logging_to_functions(original_code)
print(modified_code)
This example parses Python code, adds a logging statement to each function, and then unparsed the modified AST back into code.
Metaprogramming techniques like these allow for powerful abstractions and can significantly reduce boilerplate code. They're particularly useful for creating domain-specific languages, implementing design patterns, and building flexible frameworks. However, they should be used judiciously, as they can also make code harder to understand if overused.
These examples demonstrate how metaprogramming in Python allows you to write code that writes, modifies, or introspects other code, opening up powerful possibilities for creating flexible and dynamic software systems.
Thank you for reading! To get in touch with us, feel free to access:
- 👉 Support Page - chat with the team behind AppSeed
- 👉 Custom Development Services section and get a quote