How to use Bootstrap with Flask
Learn how to integrate Bootstrap with Flask and code production-ready web apps. Free samples included (published on Github).
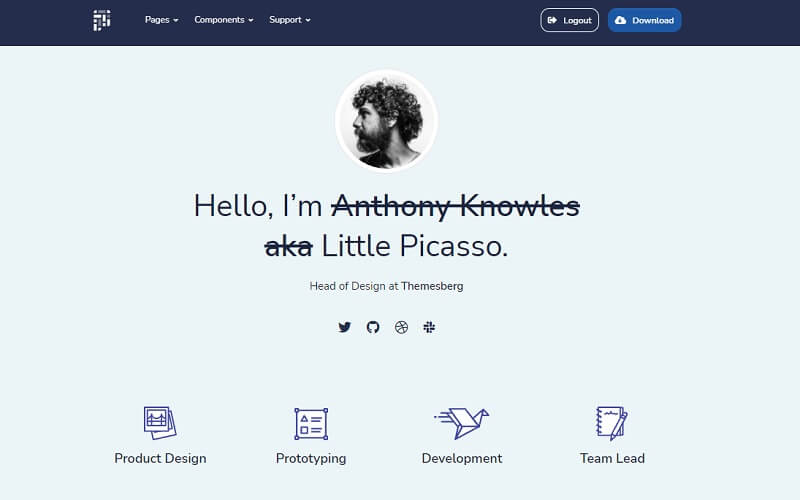
This article explains How to use Bootstrap with Flask and code professional web apps with a responsive layout. For newcomers, Bootstrap is the most popular CSS framework with more than 150k Github stars and a huge ecosystem behind it. Flask is an open-source framework used to code from simple one-page projects to complex APIs, microservices, or even complex eCommerce solutions.
In order to make this article more useful, we will take a look at an open-source Flask project that provides a beautiful UI styled with Bootstrap in two flavors: a minimum version that uses components downloaded from the official Bootstrap Samples page and a production-ready
version with more pages (home, about, contact) and a complete set of components.
Thanks for reading! Topics covered by this article:
- Section #1 - What is Bootstrap
- Section #2 - What is Flask
- Section #3 - Set up the environment
- Section #4- Code a Flask app - with authentication
- Section #5 - Integrate a fully-fledged UI Kit
- Free sample with more modules: Flask Bootstrap Pixel Lite
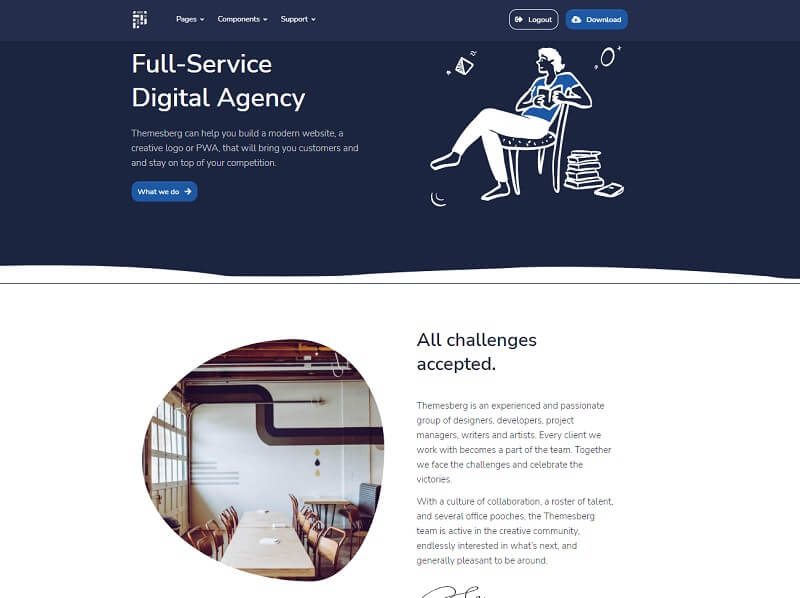
1# - What is Bootstrap
In a single sentence, Bootstrap helps us to code faster and easier user interfaces by providing a set of HTML, and CSS templates for creating UI components like buttons, dropdowns, forms, alerts, modals, tabs, accordions, etc. Reusing the assets provided by the Bootstrap framework we might win time and also improve the quality of our end-product.
Reasons to use Bootstrap
- Easy to use - any developer with basic programming knowledge can use and combine Bootstrap components to prototype fast a web page.
- Responsiveness - Bootstrap components are built to adapt and display properly on desktop and mobile devices with a wide range of resolutions.
- Alive Community - this amazing framework is actively supported and versioned by a huge open-source community
- Cross-Browser Compatibility - Bootstrap keeps up the codebase with the latest standards for a smooth deployment on all well-known browsers: Chrome, Safari, IE, or Firefox.
To getting started with Bootstrap and code a new web page is pretty easy. Just copy below snippet, save it locally and after, visualise the file using Chrome or Safari browser.
<!doctype html>
<html lang="en">
<head>
<title>My First Bootstrap Page</title>
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<h1 class="text-primary">
Get started with Bootstrap
</h1>
<!-- Bootstrap Javascripts -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Simple Bootstrap Page
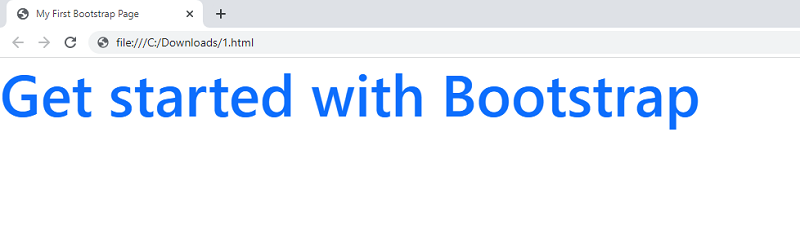
In the same way we can download more components and samples from the official website and build more complex pages with a left menu, a navigation bar, footer, and horizontal sections.
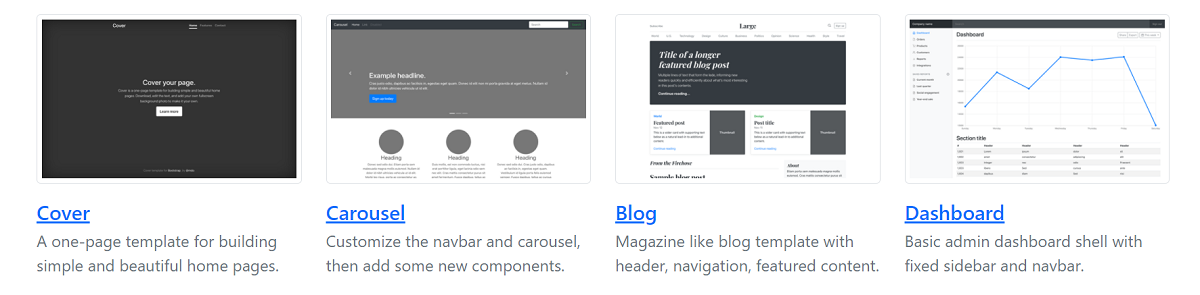
2# - What is Flask
Flask is an open-source web framework coded in Python using a lightweight codebase and flexible by design. By default, Flask is not coming with a database or any other hard dependency empowering the developers with total freedom in terms of used modules and how to structure the project. To use Flask
and code a test application, Python3 should be properly installed in the workstation and accessible in the terminal window.
$ pip install flask
The above command install Flask
using PIP
the official package manager for Python. Once Flask
is installed, we can code a simple app and run it:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Flask is great!'
if __name__ == '__main__':
app.run()
If we execute the above snippet and open http://localhost:5000
in the browser we should see the message Flask is great
. Even if not too much, we've just coded an usable Flask application that we can improve with more features and modules.
3# - Set Up Environment
To run successfully the all samples and code snippets provided in this article a minimal programming kit is required to be properly install in our workstation and fully accessible by the terminal window.
- A modern editor - VSCode or Atom
- Python3 - a modern script language used for many types of projects
- GIT - a command-line tool used to download sources from Github
VSCode - a popular and free code editor
VsCode helps us to visualize and edit the sources, execute our projects and investigate the issues that might occur during the programming process.
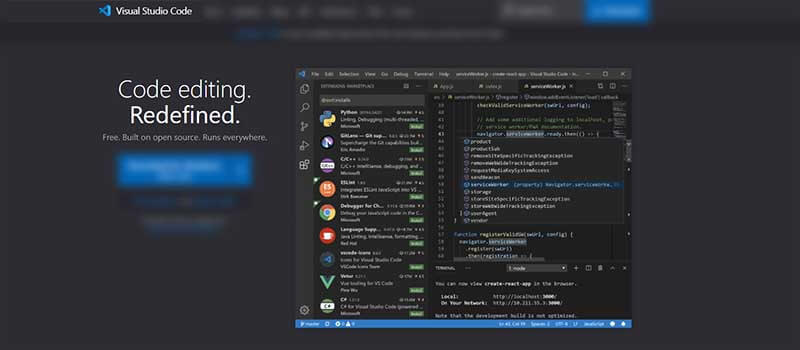
Python Programming Language
Python is a general-purpose coding language—which means that, unlike HTML, CSS, and JavaScript, it can be used for other types of programming and software development besides web development. Python is an interpreted language (translated into bytecode at runtime) and can be used for things like:
- Basic programming: using strings, adding numbers, open files
- Writing OS system scripts
- Back end (or server-side) web and mobile app development
- Desktop applications and software development
Python can be downloaded and installed from the official website with just few clicks. Once is installed, we can check the installation in the terminal:
$ python --version
Python 3.7.2
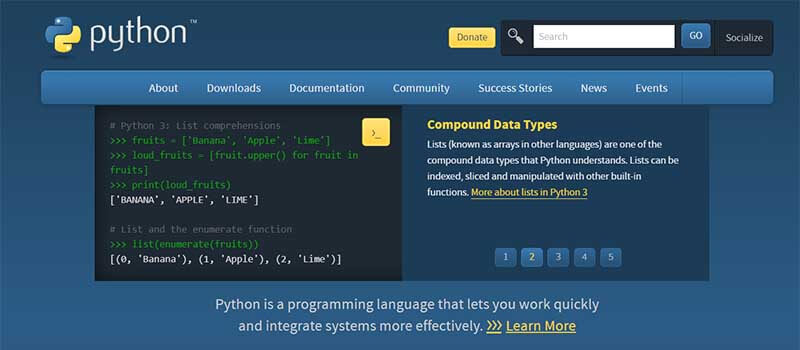
GIT command-line versioning tool
Git is a free version control system designed to handle everything from small to very large projects with speed and efficiency. Using GIT we can clone/download and manage projects from Github and BitBucket with ease.
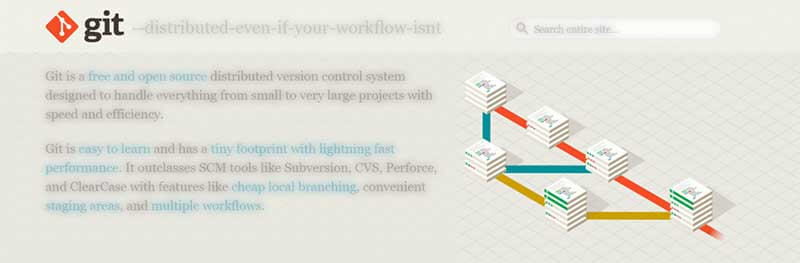
With this minimal set up we can move forward and code a simple Flask app that provides a session-based authentication and a few pages like SignIN, SignUP built with components downloaded from Bootstrap.
4# - Code Flask App (with authentication)
The project aims to be pretty simple with an intuitive codebase structure, SQLite persistence, and three pages (index, login, register) styled with Bootstrap 5. To cover all requirements a few Python modules should be installed:
Flask
- the framework that powers the appFlask-Login
- a popular library used to manage the sessionsFlask-Bcrypt
- used for password encryptionFlask-SqlAlchemy
- a popular library to access the database
Regarding the codebase structure, Flask allows up to configure the files without any constraints. Here is a possible structure that isolates the design from the functional part (routes, models) nicely.
< PROJECT ROOT >
|
|-- app/
| |-- static/
| | |-- <css, JS, images> # CSS files, Javascripts files
| |
| |-- templates/
| | |
| | |-- index.html # Index File
| | |-- login.html # Login Page
| | |-- register.html # Registration Page
| |
| |
| config.py # Provides APP Configuration
| forms.py # Defines Forms (login, register)
| models.py # Defines app models
| views.py # Application Routes
|
|-- requirements.txt
|-- run.py
|
|-- **************************************
In this simple structure, the most relevant files are listed below:
run.py
- the entry point and the value ofFLASK_APP
environment variableapp
directory bundles all files and assets used in our projectapp/config.py
- defines app configurationapp/forms.py
- definesSignIN
,SignUP
authentication formsapp/models.py
- defines the Users table (saves users and passwords)app/views.py
- handles the app routing likelogin
,logout
andregister
Another important location is the templates
directory where are defined the index
file and SignIN
, SignUP
pages. In order to use Bootstrap, the CSS and JS assets are loaded via CDN, a remote blazing-fast storage server without any local storage.
Index.html - Styled with Bootstrap
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="">
<meta name="author" content="Mark Otto, Jacob Thornton, and Bootstrap contributors">
<meta name="generator" content="Hugo 0.88.1">
<title>Flask Authentication | Bootstrap v5.1</title>
<!-- CSS only -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.1/dist/css/bootstrap.min.css">
<link rel="stylesheet" href="/static/assets/css/custom.css">
</head>
<body>
<main>
<!-- PAGE Content -->
</main>
<!-- JavaScript Bundle with Popper -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.1/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
The above code (simplified version for clarity proposes) will produce the following layout without built entirely from downloaded components. The functional part of our project detects if the user is authenticated or not and suggest to login
or register
a new account.
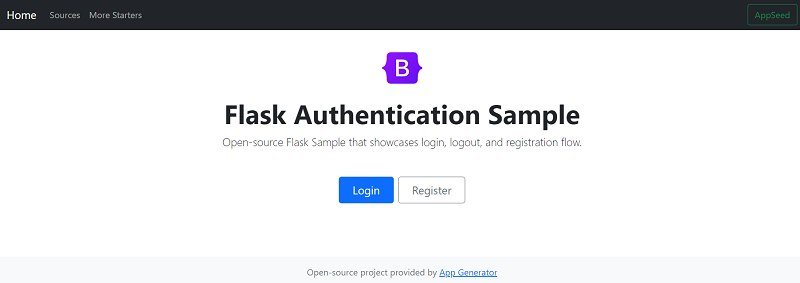
Guest users are able to create a new account via a simple page as shown below:
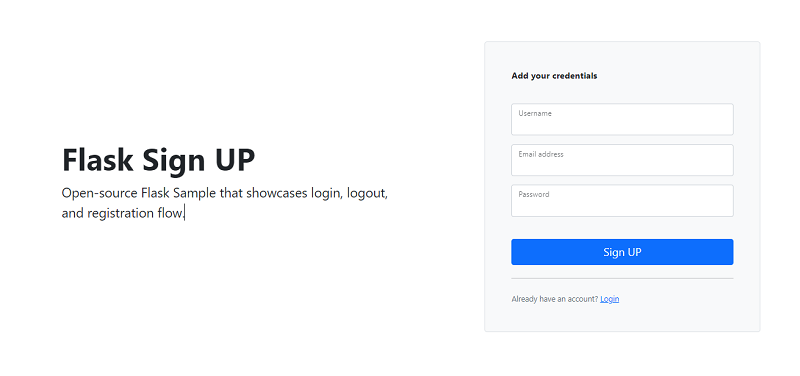
Flask Bootstrap SignIN Page
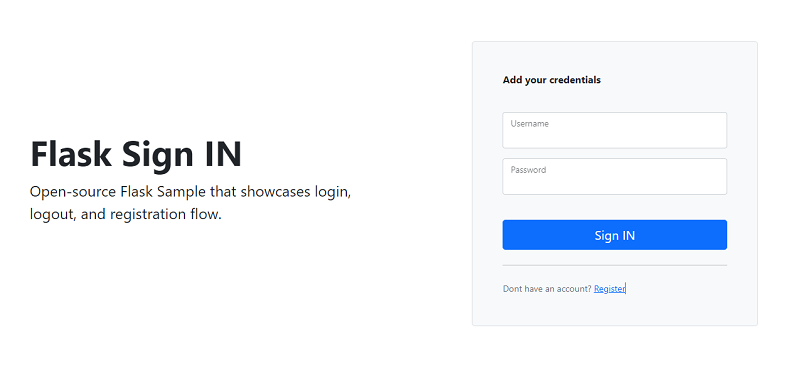
5# - Integrate Pixel Lite (free version)
Using a minimal UI kit might be ok for most projects but using a design crafted by an agency is probably better. In order to add more value to our sample we will use an open-source Bootstrap 5 design crafted by Themesberg: Pixel Lite. This amazing design comes with pre-built pages (signIn, signUp, about) and a rich set of UI components that we can use and combine. Bellow are the pages integrated into our simple project:
Flask Pixel Bootstrap Kit - Freelancer Page
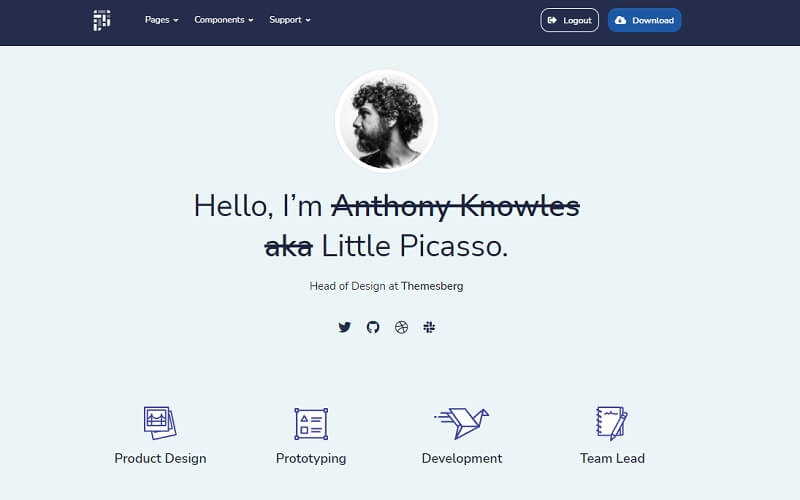
Flask Pixel Bootstrap Kit - About Page
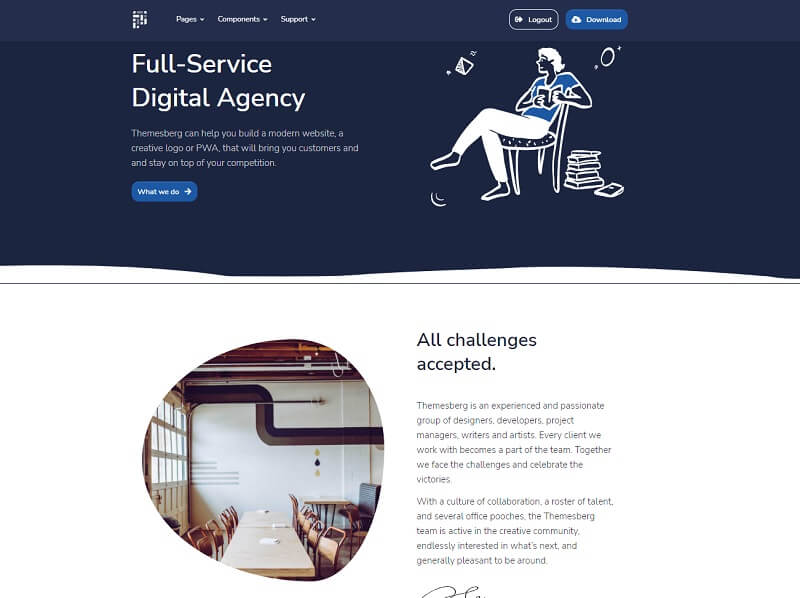
We can easily see that this new design is definitely better. Let's compile the free sample published on Github by following the build instructions provided in the README file saved at the root of the project.
Step #1 - Clone the project
$ git clone https://github.com/app-generator/flask-how-to-use-with-bootstrap.git
$ cd flask-how-to-use-with-bootstrap
Step #2 - Create a virtual environment
$ virtualenv env
$ source env/bin/activate
Step #3 - Install dependencies
$ pip3 install -r requirements.txt
Step #4 - Set Up Environment
$ export FLASK_APP=run.py
$ export FLASK_ENV=development
Step #5 - Start the app
$ flask run
By default, the application will redirect guest users to authenticate (SignIN page). Once we create a new user and sign in
, the app provides access to all private pages.
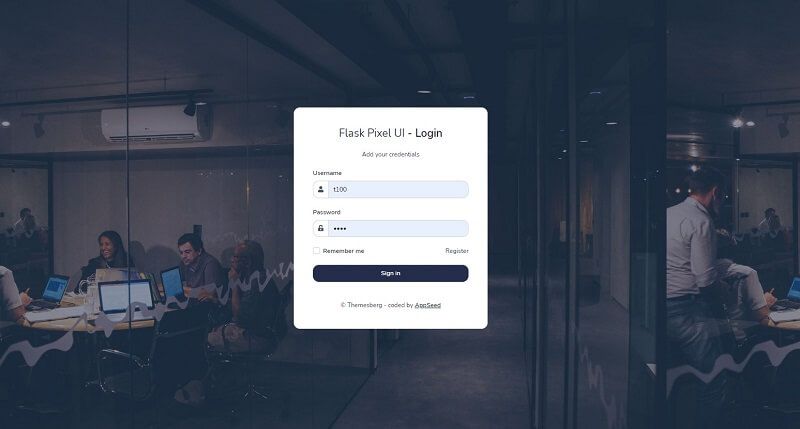
Thanks for reading! For more resources, please access:
- For support join AppSeed community on Discord
- More Flask Apps and Dashboards - free & commercial