Introduction to React Components
Get started with React and learn how to use components: create, reuse, unmount - an article by Kolawole Mangaboo
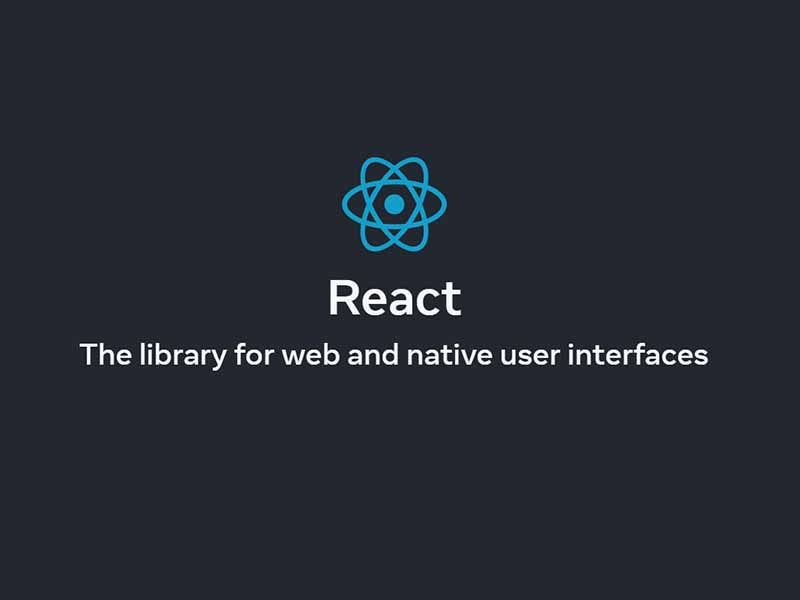
React is one of the most used libraries for frontend development in JavaScript. Being the most popular and demanded skill on frontend job applications, it can be beneficial to learn more about the technology, its architecture, and its advantages.
they
In this article, we will primarily focus on learning more about React components. You will be introduced to React architecture, the life cycle of elements, and React concepts such as props, and states.
β Introduction to React Components
In web development, a component is a piece of UI that can be reused throughout the whole application or project. In this case, a component can go from a simple text to a <p>
tag or a <button>
to a complex form that can be customized through some parameters.
But why use components? Well, they come with a lot of advantages.
- Reusability: Components can be reused in the application thus reducing code duplication and allowing the respect of the D.R.Y (Don't Repeat Yourself) rule.
- Modularity: Each component handles a specific part of the application. Working with components forces you to attribute one and only one specific goal to the code used to build this component.
- Testing: Because they are modular by default, it is easy to test components without loading the whole page or web application.
Now that we know more about UI components, their goals, and their advantages, let's dive deeper into the concept of the component but this time in React.
β Overview of React Components
React is defined as a library instead of a framework because it only deals with UI rendering and leaves many of the other things that are important in development to the developers or other tools.
To build a full working React application, you will need to install packages to manage routing through the application, complex state, your styles and much more. However, a framework based on React, Nextjs comes with everything required from web frontend framework.
In React, components are the building blocks of a user interface. They are reusable and independent pieces of code that encapsulate the logic and rendering of a specific part of the UI. Components allow you to divide your UI into smaller, manageable pieces, making it easier to develop, test, and maintain your application.
In modern React, components are written as JavaScript functions that accept properties (props) as input and return JSX (JavaScript XML) to describe the UI. Here is an example of a component in React :
import React from 'react';
const Description = (props) => {
return (
<div>
<h1>Hello, {props.name}!</h1>
<p>{props.description}</p>
</div>
);
};
export default Description;
The Description
component takes props
as a parameter which is an object that should contain a name and description properties. Using the JSX syntax, the curly braces {}
are used to embed JavaScript expressions or variables within JSX. In this case, the name
variable is included within the curly braces to display the dynamic content.
With JSX also, we can reuse the components we have defined in other React components. Here is an example.
import React from 'react';
const Greetings = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
const Description = (props) => {
return (
<div>
<Greetings name="Naruto" />
<p>{props.description}</p>
</div>
);
};
export default Description;
In the code above, we have defined a component called Greetings
that takes props
as a parameter that should contain a name property. We then use this object to display the name passed to the component.
Reading the code of a component in React, we clearly understand that the code is different from regular HTML. JSX allows you to write HTML-like code within JavaScript, making it easier to describe the structure and appearance of UI components. JSX is not valid JavaScript, but it gets transpiled into regular JavaScript by tools like Babel before it is executed by the browser.
Now that we understand how React components work, we can dive deeper into React architecture and how React works with the DOM, and the life cycle of a React component.
β Architecture of React
React uses something called the Virtual DOM, which can be compared to a lightweight copy of the actual web page or DOM. This Virtual DOM helps React make updates to the web page much faster.
So, imagine you have a component, like a button, in React. If something changes, like the text on the button, React compares the new version of the button with the old version in the virtual DOM. It figures out what exactly changed, and then only updates those specific parts in the actual web page. This process is called "diffing." By only updating what's necessary, React makes the updates faster and more efficient.
The Virtual DOM acts like a middleman between React and the DOM. It helps React keep track of what needs to be updated, and then it applies those changes all at once to the real DOM.
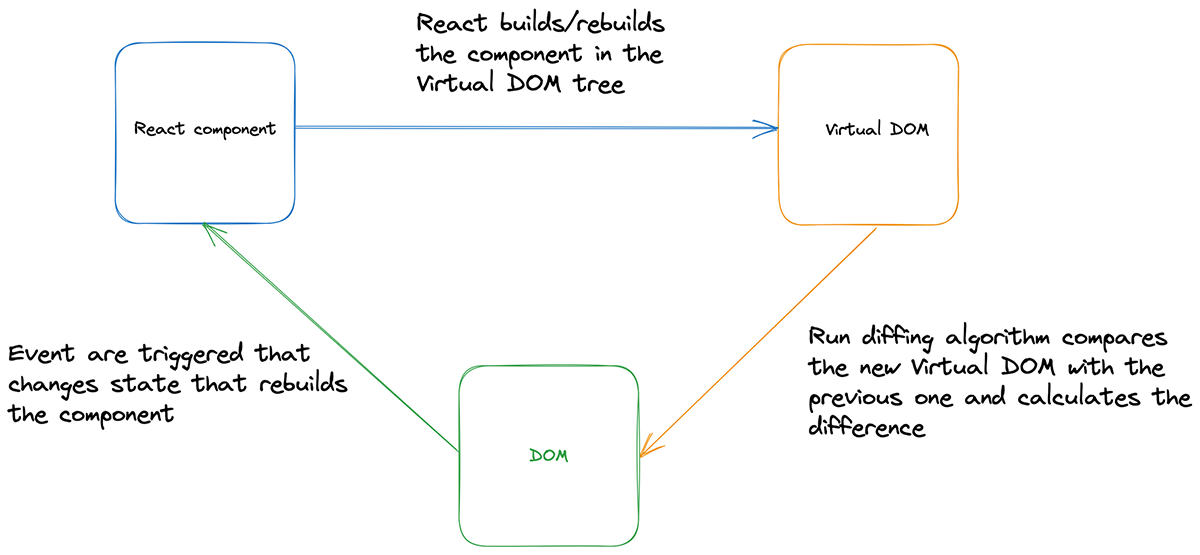
Data is actually the thing that triggers change through a web application. It is the same case for React as the modification of data will trigger effects or actions that the user can or can't see. Let's discuss state and props in React in the next section.
π Props and state
Props (short for properties) in React are used to pass data from a parent component to its child component. They allow you to customize the behavior or appearance of a child component based on the data provided by its parent.
When a parent component renders a child component, it can pass values to the child component by specifying props as attributes. The child component can access these props and use the data within its own logic or rendering.
Props in React are read-only, meaning that the child component cannot directly modify the props it receives from its parent. They are immutable and serve as a way to provide data or configuration to the child component.
In the code example to showcase the structure of a component in the Overview of React component
, the Header
component canβt modify the props
object passed as a parameter by the Description
component.
On the other hand, a state is used to manage data that can change over time within the component itself. The state represents the internal state of a component and is private to that component, meaning it cannot be directly accessed or modified by other components.
Unlike props, which are passed from a parent component and remain immutable within the child component, the state allows a component to manage and modify its own data. React provides special methods and hooks, such as useState
, to work with the component's state.
Letβs write a component that will use the Description
and Header
components to dynamically change the name of the user with a click of a button.
import React, { useState } from 'react';
const Greetings = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
const Description = (props) => {
return (
<div>
<Greetings name={props.name} />
<p>{props.description}</p>
</div>
);
};
const App = () => {
const [name, setName] = useState('Naruto');
const handleClick = () => {
setName('Sasuke');
};
return (
<div>
<Description name={name} description="This is a description." />
<button onClick={handleClick}>Change Name</button>
</div>
);
};
export default App;
useState is a Hook in React that allows you to add a state to functional components. In other words, useState
allows you to manage the state of your component, which is an object that holds information about your component and can be used to re-render the component when this state changes.
The App
component uses the useState
hook to manage the name
state variable with an initial value of 'Naruto'. Clicking the "Change Name" button triggers the handleClick
function, which updates the name
state to 'Sasuke'.
The App
component renders the Description
component, passing the name
and description
props. The name
prop is dynamically updated based on the name
state variable.
With a state changing in a component, it is clear that a component in React can go through changes. These changes can put the component in a different cycle each time but React components have a lifecycle that is important to understand.
π Life Cycle of a React Component
Understanding the life cycle of a component in React will help you write better React code depending on your needs.
- Initialization: In this stage, the component is created, and the initial state and props are set. You can use the
useState
hook to initialize the state and the component function itself to define the initial props. For example:
import React, { useState } from 'react';
const App = (props) => {
const [name, setName] = useState('Naruto');
// ...
};
2. Mounting: During the mounting stage, the component is rendered for the first time and inserted into the DOM. The useEffect
hook with an empty dependency array ([]
) can be used to mimic the behavior of the mounting stage. It will run once, similar to componentDidMount
for class components in React.
import React, { useState, useEffect } from 'react';
const App = (props) => {
const [name, setName] = useState('Naruto');
useEffect(() => {
// Mimicking componentDidMount
console.log('Component mounted');
// Cleanup function (mimicking componentWillUnmount)
return () => {
console.log('Component unmounted');
};
}, []);
// ...
};
3. Updating: The updating stage occurs when the component's state or props change. You can use the useEffect
hook with dependencies to handle side effects when specific state or prop values change. This is similar to the componentDidUpdate
lifecycle method.
import React, { useState, useEffect } from 'react';
const App = (props) => {
const [name, setName] = useState('Naruto');
const handleClick = () => {
setName('Sasuke');
};
useEffect(() => {
// Mimicking componentDidUpdate
console.log('Component updated');
}, [name]); // Run the effect only when `name` changes
// ...
};
4. Unmounting: The unmounting stage occurs when the component is removed from the DOM. You can use the cleanup function in the useEffect
hook to mimic the behavior of componentWillUnmount
. It will be executed before the component is unmounted.
import React, { useState, useEffect } from 'react';
const App = (props) => {
const [name, setName] = useState('Naruto');
const handleClick = () => {
setName('Sasuke');
};
useEffect(() => {
// Mimicking componentDidMount
console.log('Component mounted');
// Cleanup function (mimicking componentWillUnmount)
return () => {
console.log('Component unmounted');
};
}, []);
// ...
};
Here is a diagram describing a React component lifecycle.
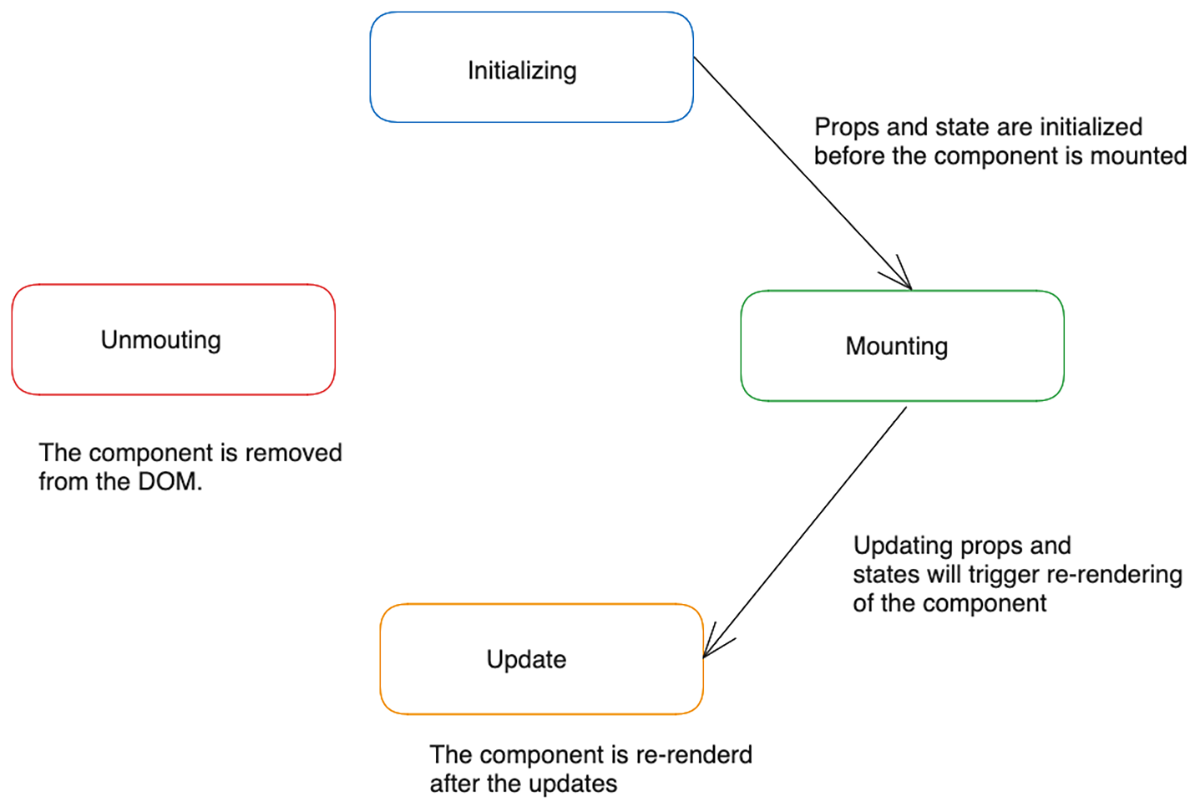
With the knowledge of React components lifecycle acquired along with state, props, and Virtual DOM, you can now say that you understand the basics of React.π The React documentation is rich and helpful if you want to dive deeper into other concepts so feel free to visit https://react.dev.
β Conclusion & Links
In this article, we have introduced the philosophy of React development and its architecture. We have talked about components, state, and props, their lifecycle, and how React used the virtual DOM to update efficiently the DOM.
Now that we understand how React works under the hood, we can learn more about React by building a form.
For more resources, feel free to acces:
- π A curated list with React Starters (free & PRO) actively supported
- π Admin Dashboards - a huge index with templates and apps
- π Custom Development Services - provided by AppSeed