JavaScript Basics and Cheat Sheet (for beginners)
A comprehensive theoretical introduction to JavaScript plus 10 items Cheat Sheet.
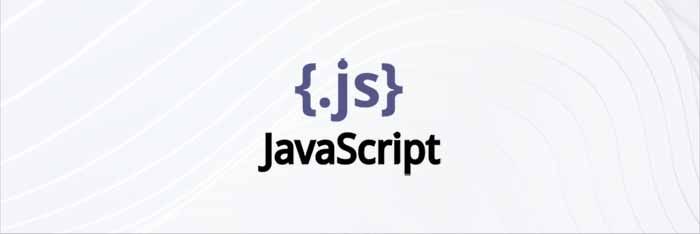
Hello! This article aims to help beginners accommodate JavaScript concepts. For newcomers, JavaScript
is known to be the most popular language in the world with over 69.7% of use in software development. If you’re going to put aside time and energy to learn a new programming language or to get your career started in tech, you’ll want to make sure you’re picking a useful and a high in-demand programming language among a lot of languages, JavaScript
is easily the best choice for you here.
Thanks for reading! Topics covered:
- 👉 What is
JavaScript
- 👉
JavaScript
Basics - 👉
Using JS
in HTML - 👉 Calling an
external JS
file - 👉
JavaScript
Comments - 👉 Commons Methodologies in
JS
- 👉
JS Cheat Sheet
- 10 items - 👉 Conclusion & Links
Now that you’ve done your research and probably kickstarted your learning, you might have found some of the concepts of JavaScript
ambiguous and difficult to wrap your head around; don’t worry, we’ve all been there and that is why we’re putting together this article to help you learn and understand the basics of this awesome language while also giving you career-advancing cheat sheets to take your JavaScript mastery to the next level.
Already Familiar with JS?
Check out this popular article: React Forms - Formik vs. Hook-Form vs. Final-Form
This article is for beginners and entry-level engineers who are looking to advance their JavaScript skillset and understanding of the language. This article contains explanations, example code, basic and important operators, functions, methods, classes, and a general overview of the basic principles of JavaScript
.
✨ What is JavaScript
JavaScript in the simplest form is a programming language that powers most dynamic behaviors on a website because it has APIs that work with text, data structures, and Document Object Model (DOM). It was developed in 1995 and it is a high-level multi-paradigm scripting language that supports functional, imperative, and event-driven programming styles.
Some of the uses of JavaScript include:
- Loading of web page content via WebSockets or Ajax.
- Initialize pop-ups, queries, and manipulate elements
- Control streaming media playbacks, e.t.c.
Popular websites that run on JavaScript include Google, Facebook, Youtube, Instagram, and so much more. This makes it the most used language in the history of the internet. Owing to this fact, there’s just so much to learn and it’s impossible to know all the concepts of the language memorized in your head which makes this article very important for your growth as a JavaScript developer.
✨ JavaScript Basics
Here are some basic concepts in JavaScript you should be familiar with:
👉 Including JavaScript
in an HTML page
To include JS in an HTML page, you’ll need to wrap the code in <script> tags. Here's an example of this concept:
<script type="text/javascript">
//Your JavaScript code goes here
</script>
👉 Calling an External JavaScript
file
You can create an external JavaScript file and link to it in your HTML file. This is useful because your JavaScript file can have a lot of code within it and populating this in your HTML file would make the code look terrible and out of place. So if your file is named script.js
, here’s how to call it in your HTML file.
<script src="https://somehost.net/static/myscript.js"></script>
👉 Commenting Your Code
Comments are necessary for your codebase because they help other people to understand it and also help you to debug your code when it's needed.
There are different ways to add comments to your JavaScript code. They include:
- Single-line comments: Use the “//” as the preceding characters to add single-line comments to your codebase.
// Single line comment in JS
let my_var = "Learning JS"
- Multi-line comments: This is useful when you want to write multi-line comments in your code. Wrap your comments in /* and */ as the start and finish of your comment to avoid it being executed.
/*
Multi line comment in JS
*/
var x = 4;
✨ Common JS Methodologies
👉 Methods
Methods return information about an object, and are called by appending an instance with a period *.*, the method name, and parentheses ().
// Returns a number between 0 and 1
Math.random();
👉 Libraries
Libraries contain methods that can be called by appending the library name with a period *.*, the method name, and a set of parentheses (). Here is an example of a library in JavaScript.
Math.random();
// ☝️ Math is the library
👉 Variables
Variables are used to store information in JavaScript. It is a unique identifier that points to a specific location in memory. Variables are stand-in values that can be used to perform various operations in JavaScript codebases and you should note that there are 3 common ways by which you can declare variables in JavaScript. They include:
- var - This is the most commonly used JavaScript variable. However, due to its redeclaration possibility, i.e. it can be easily redeclared and redefined within the code which makes it highly unstable and prone to errors, therefore it’s been advised to not use the var keyword constantly inside your codebase since the adoption of ES6’s let keyword.
- let - The let keyword variable cannot be redeclared, making it prone to fewer errors. They can, however, be reassigned a new value.
// Note here that ‘x’ cannot be redeclared
// but can be reassigned as a value
let x = 5;
- const - The
const
variables in JavaScript cannot be used before they appear in the code. They also cannot be redeclared or reassigned value, i.e. the information they store in memory remains constant throughout your code lifetime.
// y here remains constant throughout the codebase
// and cannot be reassigned or redeclared.
const y = 15;
👉 Data Types
Different types of values and data can be stored in JavaScript variables. The “=” equal to sign is used to assign values to variables. There are 7 types of data types in JavaScript including:
- Numbers - These are numerical values in JavaScript which can be either integers or real numbers
//10 here is a numerical data type.
let x = 10;
- Strings - These are a combination of multiple characters in JavaScript. An example of a string in JavaScript would be:
let name = “Hello JavaScript”
- Boolean - These are truthy or falsy values in JavaScript.
- Array - An array is a collection of different data types, i.e., you can store various kinds of data types within an array. It is usually represented with a bracket []. An example of an array is:
let mango = [‘fruit’, true, 10]
- Object - An object in JavaScript is a container for the properties of a named variable. They can have their data and methods. e.g.
let police = {name: “Jon Snow”, id: 5667637, active: true}
- Null - This is used to represent the absence of identity, and can only come from literal expressions e.g.
let x = null
It is often used to indicate that a value is expected but might be unavailable at this time.
✨ JavaScript Cheat Sheet
👉 1# - Operators in JavaScript
JavaScript operators are used to assign values, compare values, perform arithmetic calculations, and so much more. There are different kinds of operators in JavaScript including arithmetic operators, logical operators, comparison operators, and bitwise operators.
- Arithmetic Operators
These are used to perform basic calculus in JavaScript:
+ Addition
- Subtraction
* Multiplication
/ Division
(....) Grouping Operator
% Modulus
++ Increment Numbers
-- Decrement Numbers
- Comparison Operators
== Equal to
=== Equal value and equal type
!= Not equal
!== Not equal value or not equal type
> Greater than
< Less than
>= Greater than or equal to
<= Less than or equal to
? Ternary operator
- Logical Operators
&& Logical and
|| Logical or
! Logical not
- Bitwise Operators
& AND statement
| OR statement
~ NOT
^ NOR
<< Left shift
>> Right shift
>>> Zero fill right shift
👉 2# - Conditional Flow (if/else)
These statements are used to perform tasks and commands within your code. They dictate the flow of commands your codebase is going to execute, i.e., if certain requirements are met, then your code executes or not, which makes it an important aspect of JavaScript and programming in general.
if ( condition ) {
// block of code to be executed
// if the given condition is satisfied
} else {
// block of code to be executed
// if the given condition is not met
}
👉 3# - Loops in JavaScript
Loops are similar to “if-else” statements because they run particular commands as long as a condition is met, however, the major difference is that loops let you run code blocks as many times as you like with different values. There are different ways to create loops in JavaScript which include:
- The while loop: This establishes a condition under which a loop will run. Here is an example of the syntax of a loop:
while ( condition ) {
// Code to be executed
// Update the loop variable
}
- For loop: This is the most popular method for creating a loop in JavaScript. Its syntax is shown below:
for (initialize loop variable; condition; update after loop) {
// code to be executed in loop
}
- The do-while loop: This is very similar to the while loop, but the difference here is it runs at least once and checks to the end to ensure that the condition is met before running again. Its syntax is shown below:
do {
// 1. Code to be executed in the loop
// 2. Update loop variable
} while (condition);
👉 4# - JavaScript Arrays
Arrays are important data structures in JavaScript that are used to group small or large sets of data. Arrays are simply known as a collection of data. An example of an array is shown below:
let array = [“goodness”, “maya”, “job”];
Arrays have special methods used to perform specific operations on the data contained within them, they include:
concat()
- Used to join several arrays together into one.length()
- This indicates the number of elements contained within an array.indexOf()
- This returns the first position at which a given element contained in an array appears.join()
- The join method in JavaScript is used to combine elements of an array into a single string and also return the string.lastIndexOf()
- Gives the last position at which a given element is present in an array.pop()
- This removes the last element of an array.push()
- This adds a new element at the end.reverse()
- This sorts elements of an array in descending order.shift()
- This method removes the first element of an array.slice()
- This pulls a copy of an array into a new array.sort()
- This method arranges elements of an element alphabetically.splice()
- This adds elements in a specified way and position.toString()
- Converts elements of an array to strings.unshift()
- This method adds a new element to the beginning of the array.valueOf()
- Returns the primitive value of the specified object.
👉 5# - JavaScript Functions
Functions in JavaScript can be defined as chunks of code written to perform a specific set of instructions. The following describes the syntax of a basic function in JavaScript:
function myFunction (parameterOne, parameterTwo, …parameterN) {
// Task of the function
}
The code block above begins with the keyword function
which is used explicitly to describe a function in JavaScript, followed by the name of the function, and the parameters of the function defined within the parenthesis ()
, and finally followed by the task/job the function is supposed to undertake in curly braces {}
.
Listed below are some popular types of functions used for outputting data in JavaScript
:
prompt()
: This function creates a dialogue box that takes a user’s input within the browser.alert()
: This function outputs information in a dialogue box in the browser’s window.console.log()
: This is a very important function for developers of all levels because it is used for debugging code and also outputting information in the console of browsers.document.write()
: This function is used for writing code directly into an HTML code.
Below are some Global functions in JavaScript:
parseFloat()
: This function returns a floating-point number of the argument passed to it.parseInt()
: This returns an integral number of any argument passed to it.encodeURI()
: Encodes a Uniform Resource Operator (URI) into the UTF-8 encoding scheme.decodeURI()
: Decodes an already encoded URI.isNaN()
: This function determines whether an argument given to it is a number or not.Number()
: Used for converting a given argument to a number.eval(): Used for evaluating JavaScript programs presented as strings.
👉 6# - Scope in JavaScript
Scope is defined as the accessibility of defined variables in JavaScript, i.e., it describes which section of your JavaScript code can access and use already-defined variables. In JavaScript, there are three (3) types of scope namely:
- Global Scope - This refers to any defined variable that is not contained within a code block (a pair of curly braces) or a function. The global scope can be accessed from anywhere within the program. An example of a globally scoped variable is shown below:
let greeting = ‘Hello!’;
function greet() {
console.log(greeting);
}
// This function returns ‘Hello!’ as the response.
greet();
- Local Scope - These are variables declared within a function block. They are only accessible within the function and cannot be used elsewhere within the program. Below is an example of local scope:
function greet() {
let greeting = ‘Hello!’;
console.log(greeting);
}
//This function returns ‘Hello!’ as the response.
greet();
- Block Scope - Variables defined with the let and const keyword can be scoped to the nearest curly brackets in ES6. They can’t however be reached from outside the said pair of curly braces, meaning they can’t be accessed outside. Below is an example of a blocked scope:
{
let greeting = ‘Hi’;
var language = ‘English’;
console.log(greeting); // “Hi” is returned
}
console.log(language); // ‘English’ gets logged
console.log(greeting); //Uncaugt ReferenceError: greeting is not defined
👉 7# - JavaScript Hoisting
Hoisting is a JavaScript methodology that allows functions, variables, and classes to be moved to the top of their scope, i.e., functions, variables, and class declarations can be utilized in JavaScript code before defining them using hoisting. This method is however not recommended as the method is most times prone to errors and unforeseen mistakes in the program.
The two types of hoisting that are available in JavaScript include:
- Function Hoisting - As earlier stated, function hoisting gives you the ability to call or use a function in your program before defining it. An example is shown below:
// The usage is before the definition
showAnimal(‘lion’)
function showAnimal(input) {
console.log(input);
} //Lion gets logged
- Variable Hoisting - Hoisting of variables allows you to utilize a variable before it is defined. It should, however, be noted that JavaScript hoists only declarations and not variable initialization.
👉 8# - JavaScript Strings
Strings are a combination of characters that are used to perform some sets of tasks in JavaScript. Strings are equipped with many methods that make it possible to perform various sets of instructions within programs. Some of these methods are listed below:
toLowercase()
- Used for converting strings to lower case.toUppercase()
- Used for converting strings to upper case.charAt()
- This method is used for returning a specific character at a given index of a string.charCodeAt()
- This method is used for returning the Unicode of a character at a given index.concat()
- Used for joining multiple strings together into one.match()
- Used for retrieving matched strings of a string against a provided pattern.replace()
- This method is used for finding and replacing a given text in a string.indexOf()
- This method is useful in providing the index of the first appearance of a given text inside a string.lastIndexOf()
- Similar to the indexOf() method, the only difference here is that it returns the last occurrence of the character and searches backward.search()
- This method is used for initiating a search for a matching text and returning the index of the searched string.substr()
- This method provides a substring from the index of a character in a string.slice()
- This method is used for extracting an area of a given string and returning it.split()
- Used for dividing a string object into an array of strings at a particular index.substring()
- This method is similar to the slice() method, only that it does not allow negative positions.valueOf() - Used for returning the primitive value of a String object.
👉 9# - JavaScript Browser Objects
This is one of the most useful JavaScript applications in programming. These browser objects make it possible for JavaScript to take note of a user’s browser activities and incorporate its properties into code, in addition to HTML elements.
Below is a list of JavaScript Window properties that are popularly used:
history
- This provides the history of the Window object.innerHeight
- Describes the inner height area of a window object.innerWidth
- Provides the inner width of a window object.pageXOffset
- Describes the number of pixels offset from the center of the page horizontally.pageYOffset
- Same with the pageXOffset property too, but scales vertically instead.document
- Returns the window’s document object.location
- Returns the window’s location object.name
- This property sets or retrieves a window’s namescreen
- Returns the window’s screenobject.self
- Returns the currently opened window.
JavaScript browser methods that are common are listed below:
alert()
- This method shows a message and an OK button in an alert box.setInterval()
- This method is used to call a function declaration or expression at regular intervals defined in your program.setTimeout()
- This calls a function or an expression after a set interval of time.clearInterval()
- Removes an interval that was set by the setInterval() method.clearTimeout()
- Removes an interval that was set by the setTimeout() method.open()
- Creates a new browser object.print()
- Prints the current window’s object.stop()
- This stops the window from opening.prompt()
- Shows a dialogue box asking for some feedback from the user.
👉 10# - JavaScript Error Handling
There are various kinds of errors that occur in the process of running JavaScript code. There are six types of errors that are returned in JavaScript:
EvalError
- This indicates when there was an error that occurred within the eval() method.RangeError
- Useful with numbers. It indicates whether a number is “Out of range”.ReferenceError
- It indicates whether there was a wrong reference within your program.SyntaxError
- Indicates a wrong syntax within your program.TypeError
- Indicates the occurrence of a type error.URIError - Indicates that an encodeURI() error was occurring.
👉 11# - JS Document Object Model (DOM)
The Document Object Model describes the structure of a webpage’s code. In JavaScript, there are several ways by which to build and alter HTML elements referred to as Nodes.
Node Properties - Below are some common JavaScript DOM nodes
attributes
- This gets the live list of all the characteristics associated with an element.baseURI
- This property returns the element’s absolute base URL.childNodes
- Returns a list of all children's nodes of an element.firstChild
- Returns an element’s first child node.lastChild
- Returns an element’s last child node.nodeName
- Returns the name of the node.nodeType
- This returns the node’s type.nodeValue
- Sets or returns a node’s value.parentNode
- Returns the element’s parent nodetextContent
- Sets or returns a node’s and its descendants’ content.
Node Methods: These methods allow JavaScript to manipulate nodes in the DOM:
appendChild()
- This method adds a new child node as the last child node to an element.removeChild()
- This removes a child node from an element.replaceChild()
-This replaces a child node in an element.normalize()
- This joins neighboring nodes together in an element.isEqualNode()
- Determines whether two nodes are equal.isSameNode()
- Determines whether two elements belong to the same node.
✨ Conclusion & Links
JavaScript is a universal language in programming with various uses in different industries and specializations and learning the language can take you from junior level engineer to senior level in a couple of years with good practice and consistency.
For more resources and tuts, feel free to access:
- 👉 JavaScript concepts for React Beginners
- 👉 NodeJS for Beginners - Practical Guide
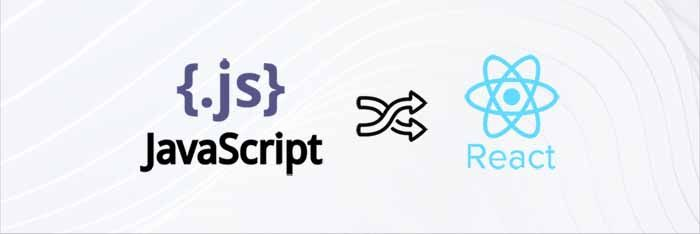