JavaScript and Vue - Short Introduction for Beginners
A comprehensive introduction to Javascript and VueJS for beginners - With Free Samples.
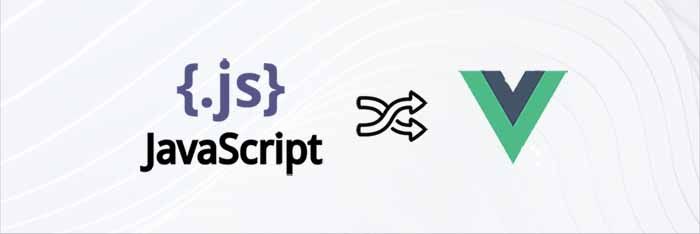
Hello! This article aims to help beginners to accommodate JavaScript and VueJS basic concepts and patterns. At the end of the article, a few VueJS samples are mentioned (all free and open-source). For newcomers, VueJS
is a leading frontend technology used to code interactive user interfaces powered by JavaScript
.
Thanks for reading! Topics covered:
- π What is
Javascript
- π
Short introduction
to JS - π JS
Variables
, Data Types, Functions, Arrays - π
VueJS Concepts
Β - π Β
Ternary Operator
,Destructuring
,Spread Syntax
- π Β
Open-Source
VueJS Samples
β¨ What is JavaScript
JavaScript has become a really hot topic in the programming language. It's not surprising because JavaScript completed several years in a row as the most used programming language.
Technically, why JavaScript is so powerful as a programming language is because Javascript core can run in all major web browsers and cross-platform over Windows, Linux boxes, or macOS via NodeJS engine. Β
Javascript is well known for the development of web apps but JavaScript can be used for a variety of purposes also: for native Mobile Applications we can use frameworks like React Native or Ionic, for Back-end Web Development we can use NodeJS, or Machine Learning.
β¨ JavaScript Introduction
To be proficient in JavaScript and be able to implement it in a web browser, you need to start learning the basics of JavaScript like variables
, data types
, arrays
, functions
, looping and control structures. Here is some basic JavaScript concepts and helpers you should know:
π JavaScript Helper Β - Console.log()
Β
This utility function is used to inspect the execution and the output of Javascript code.
<!DOCTYPE html>
<html>
<body>
<h1>Basic Javascript</h1>
<p>To open the console (Press F12).</p>
<script>
console.log('Hello.')
</script>
</body>
</html>
π JavaScript Variables
Variables are used to store information and be referenced in a program. In JavaScript, we can use var
, let
and const
keywords. If you want the value cannot be changed, use const
but if the value of the variable can change use let
.
<!DOCTYPE html>
<html>
<body>
<h1>Basic Javascript</h1>
<p>To open the console (Press F12).</p>
<script>
const number1 = 1;
let number2 = 2;
console.log('Hello.');
console.log(number1);
console.log(number2);
</script>
</body>
</html>
π JavaScript Data Types
Data types describe the different types of data that gonna be working with and stored in variables. Here is a shortlist of data types in JavaScript: String
, Number
, Array
, Object
and boolean
.
<!DOCTYPE html>
<html>
<body>
<h1>Basic Javascript</h1>
<p>To open the console (Press F12).</p>
<script>
// Number
let one = 11;
// String
let myname = 'James';
// Boolean
let isExist = true;
</script>
</body>
</html>
π JavaScript Functions
The function is a set of statements that performs a task or calculates a value and can repeat to run many times.
<!DOCTYPE html>
<html>
<body>
<h1>Basic Javascript</h1>
<p>To open the console (Press F12).</p>
<script>
function myFunc() {
return 'hello';
}
</script>
</body>
</html>
π JavaScript Arrays
The array is a special variable that can be enabled to store a collection of multiple items under a single variable name.
<!DOCTYPE html>
<html>
<body>
<h1>Basic Javascript</h1>
<p>To open the console (Press F12).</p>
<script>
const my_array = [1, 2] // this is an array type
</script>
</body>
</html>
β¨ JS Concepts used in VueJS
Before using VueJS, is recommended to study and understand in deep a few JS concepts widely used in VueJS. Let's mention a few JS concepts that help developers to be proficient while coding VueJs projects.
π JavaScript - Conditional (ternary) Operator
In VueJs, there will be many conditions to conditionally show or hide elements or text using an if...else
statement that looks less concise. The ternary operator
works exactly the same as the if statement, but is shorter and more beautiful declarative.
// If / Else version
let value = '';
const numb = 2;
if(numb === 2) {
value = 'number 2'
} else {
value = 'another number'
}
// Ternary Operator Version
value = numb !== 2 ? 'another number' : 'number 2'
console.log(value) // number 2
π JavaScript - Object Property Value Shorthand
This is for defining an object whose keys have the same name as the variables passed in as properties, you can use the shorthand. It makes your code shorter.
let one = 1;
let two = 2;
let three = 3;
// Old Way
const _object = {
one: one,
two: two,
three: three,
}
// New way
const object = {
one,
two,
three,
}
π JavaScript - Destructuring
Destructuring is a mechanism that allows extracting array or object values ββwith ease.
// Object
const student = {
name: 'James',
age: 5
}
// Destructuring Object
const { name, age } = student;
// Array
const people = ['James', 'Steve']
// Destructuring Array
const { first, second } = people;
π JavaScript - Spread Syntax
The three dots ...
syntax can be thought of as a kind of a collection
syntax where it operates on a collection of values or an iterable.
// Object
const student = {
name: 'James',
age: 5
}
const student2 = {...student};
// Array
const people = ['James', 'Steve']
const people2 = [...people];
π JavaScript - Nullish Coalescing Operator
// Classic Check
const value = 5;
const a = value !== null ? value : 0;
console.log(a); // 5
// Nullish coalescing
const b = value ?? 0;
console.log(b) // 5
π JavaScript - Arrow Functions
The arrow functions are an alternative to a traditional javascript function expression and they do have a few semantic differences. The primary benefit of arrow functions is their short syntax. We can use several shorthands in order to write our functions to remove unnecessary boilerplate, such that we can even write it all on a single line.
// regular
function addTwo(a) {
return a + 2
}
// arrow function
const addTwo = a => a + 2
// regular
function multiply(a) {
return a * a
}
// arrow function
const multiply = a => a * a
// regular
function getTwo() {
return 2
}
// arrow function
const getTwo = () => 2
π JavaScript - Import & Export
If you're working on a modern Javascript application, there are big chances that your code will be split into several files to perform certain tasks. This is a good idea because your code will be more organized and reusable in other functions. This concept is fully applicable in Vue because of the native component-based architecture of Vue.
Source File Sample - That exports
the information
// file1.js
export default function devide(a, b) {
return a / b
}
Import File Sample - That reuse exported data
// file2.js
import { devide } from './file1.js'
console.log( devide(6, 2) ) // 3
π JavaScript - Array Methods
The Array enables storing a collection of multiple items in a single variable name and I probably use this method the most frequently like:
- map() - Β creates a new array populated with the results of calling a provided function on every element in the calling array.
- filter() - creates a new array with all elements that pass the test implemented by the provided function.
- reduce() - executes a user-supplied "reducer" callback function on each element of the array, in order, passing in the return value from the calculation on the preceding element.
- find() - returns the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function,
undefined
is returned. - includes() - determines whether an array includes a certain value among its entries, returning
true
orfalse
as appropriate.
β¨ VueJS - Short Introduction
Vue is one of the most popular JavaScript front-end frameworks known as a progressive front-end framework. It's a component-based framework built on top of standard HTML, CSS, and JavaScript, which means that we can build applications with Vue by nesting components and passing data in between them.
The next sections will present a few basic concepts that might improve developers' proficiency while coding VueJS apps.
π VueJs Text Interpolation
The basic form of data binding in VueJs is text interpolation using the Mustache
syntax (double curly braces). It's will be replaced and updated whenever the value changes.
<h4>Your name: {{ yourName }}</h4>
π VueJs Β Conditional Render
We can render items on the screen by using the v-if
directive. V-if
directives are Vue codes that we can apply to make an element do something example like show or hide the element.
<h4 v-if="yourName">Your name: {{ yourName }}</h4>
π Accept Input with V-model
In VueJs can take inputs via the v-model
directive. It's called two-way binding
on a form input element or a component. With V-model
we can get and sets data to an element.
<input type="text" v-model="search" placeholder="Search..." />
π Event Handling in VueJS
VueJs handles JavaScript events very efficiently. Vue provides v-on
directive that can be replaced with @
. For example, v-on:click
is the same as with @click
. Here is some example the other v-on
directives like v-on:stop
, v-on:prevent
, v-on:self
, v-on:capture
, v-on:once
and v-on:passive
.
<button @click="doReset">Reset</button>
Those that patiently follow up on the content, can play with a few open-source VueJs Starters and Templates mention in the next section.
β¨ Vue Soft UI Dashboard
Vue Soft UI Dashboard is built with over 70 frontend individual elements, like buttons, inputs, navbars, nav tabs, cards, or alerts, giving you the freedom of choosing and combining.
- π Vue Soft UI Dashboard - LIVE demo
- π Vue Soft UI Dashboard - Product page
This Free Bootstrap 5 & VueJS 3 Dashboard is coming with prebuilt design blocks, so the development process is seamless, switching from our pages to the real website is very easy to be done.
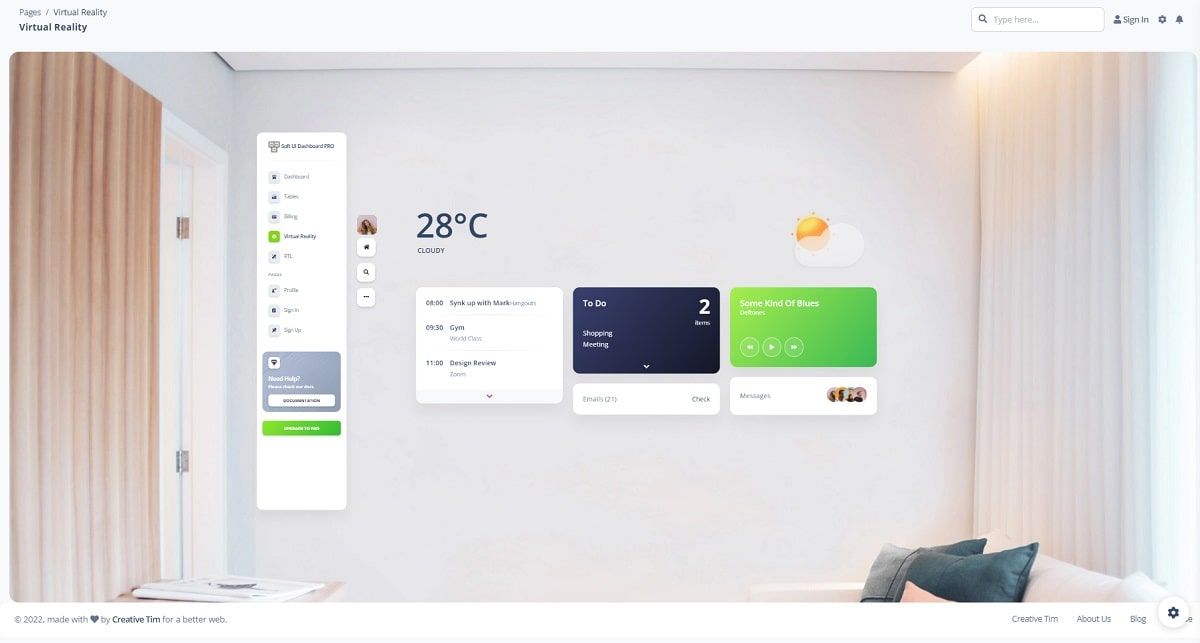
β¨ Vue Material Kit
Vue Material Kit is a beautiful resource built over Vue Material and Vuejs. It will help you get started developing UI Kits in no time. Vue Material Kit is the official Vuejs version of the Original Material Kit. Using the UI Kit is pretty simple but requires basic knowledge of Javascript, Vuejs, and Vue Router.
- π Vue Material Kit - LIVE demo
- π Vue Material Kit - Product page
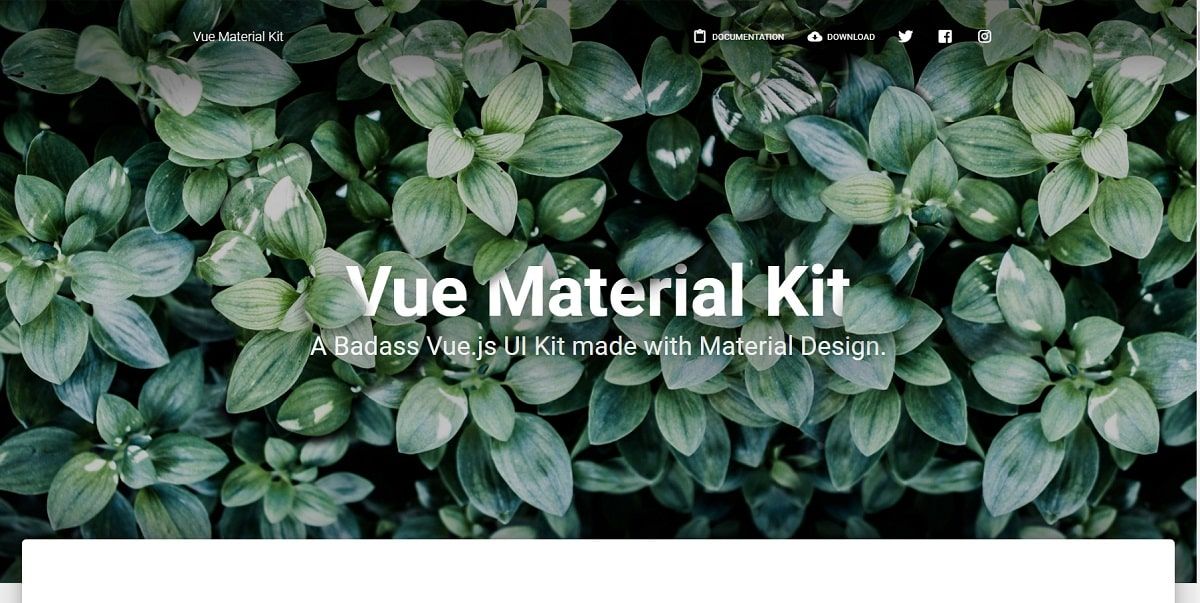
β¨ Vue Material Dashboard
Vue Material Dashboard is a free Admin Template based on Vue3 & Bootstrap5. If youβre a developer looking to create an admin dashboard that is developer-friendly, rich with features, and highly customizable, here is your match.
- π Vue Material Dashboard - LIVE demo
- π Vue Material Dashboard - Product page
This innovative Vue3 & Bootstrap5 dashboard comes with a beautiful design inspired by Google's Material Design and it will help you create stunning websites & web apps to delight your clients.
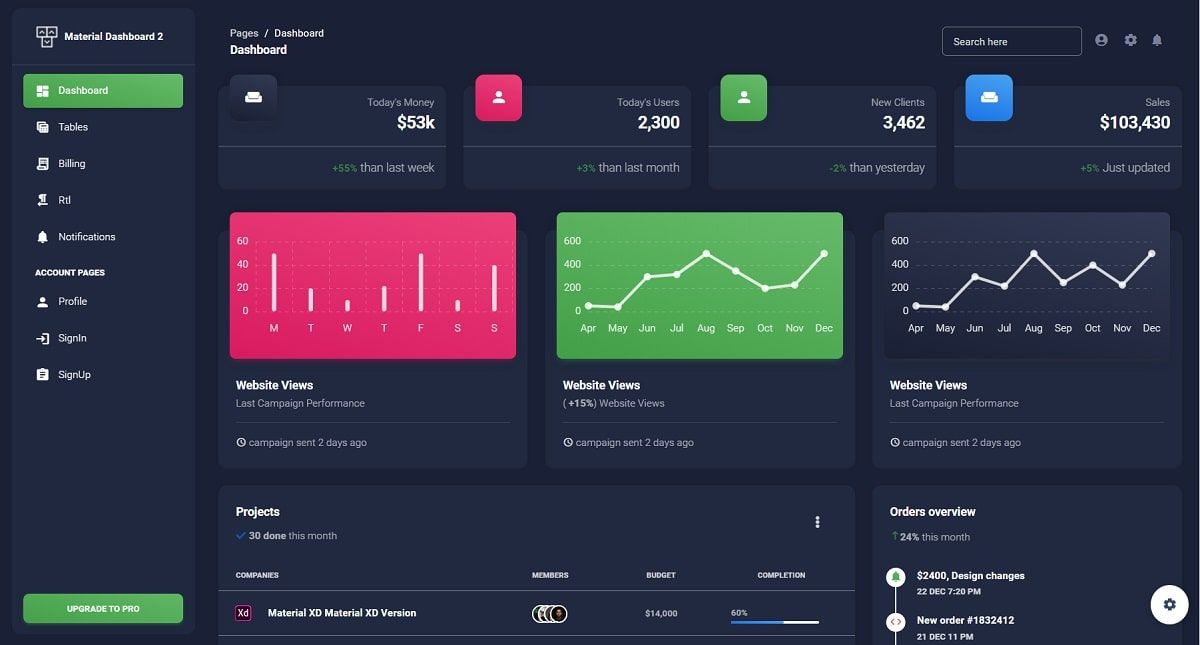