Python - Execute Javascript, C++, easily
Learn how to execute C++ and Javascript code in a Python-based program with ease.
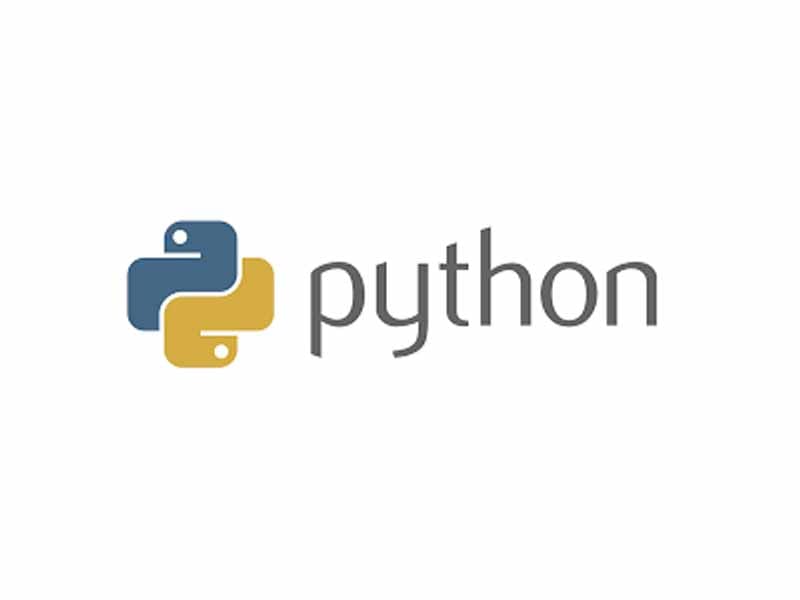
Hello Coders! This article explains how to execute code written in different programming languages like Javascript, C++, or GO in a Python-based program. The concept might help when the native language (Python in this case) becomes limited in some critical context and a better solution is to mix code from another language. Thanks for reading!
Executing JavaScript Code
You can execute JavaScript code within Python using the execjs
library. Here's a simple example:
import execjs
js_code = """
function add(x, y) {
return x + y;
}
"""
# Create a context
ctx = execjs.compile(js_code)
# Call the JavaScript function
result = ctx.call("add", 3, 4)
print(result) # Output: 7
Executing C++ Code
To execute C++ code in Python, you can use the ctypes
module or the subprocess
module to run a compiled C++ executable. Here's an example using subprocess
:
import subprocess
# Compile your C++ code first, e.g., with g++
# g++ -o my_program my_program.cpp
# Run the compiled executable
result = subprocess.run(["./my_program"], capture_output=True, text=True)
print(result.stdout)
Executing Go Code
You can execute Go code in Python by calling the Go executable or by embedding Go code in a C extension and then calling that extension from Python. Here's an example using the subprocess
module:
import subprocess
# Create a Go source file
go_code = """
package main
import "fmt"
func main() {
fmt.Println("Hello, Go!")
}
"""
with open("hello.go", "w") as f:
f.write(go_code)
# Compile and run the Go code
result = subprocess.run(["go", "run", "hello.go"], capture_output=True, text=True)
print(result.stdout)
Conclusion
Remember, these examples demonstrate simple ways to execute code in other languages from Python. Depending on your requirements, you may need to explore more advanced techniques or libraries specific to each language for deeper integration.
Also, ensure you have the necessary compilers and interpreters installed for each language you intend to execute code in.
Thanks for reading! For more resources, feel free to access:
- 👉 AppSeed, a platform already used by 8k registered developers
- 👉 Build apps with Django App Generator (free service)