React Forms - Formik vs. Hook-Form vs. Final-Form
A fair comparison over three top-rated Forms Library for React: Formik, React-Hook-Form, and React-Final-Form - With code samples and validation.
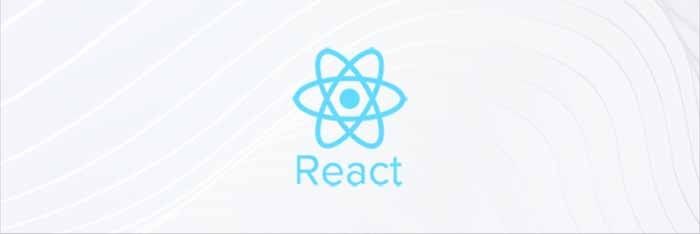
Hello! Forms are a crucial part of every application, from signing up, logging in, or collecting a survey from our users. Forms may seem simple but they are not. This article aims to provide a comparison of the top most-used React Forms with pros, cons, and code samples: Formik
, React Hook Form
and React Final Form
. For newcomers, React is a super popular JS library for coding interactive user interfaces baked by Facebook. Thanks for reading!
🚀 PROMO
In case you're a junior developer or know one, this PROMO Bundle crafted, and Discounted with 85% by Creative-Tim might be useful. The package includes a rock-solid collection of premium assets (Kits & Dashboards) that can be used to build eye-catching portfolios and web apps in no time.
👉 Junior PROMO Bundle - 24 PREMIUM Kits & Designer Files
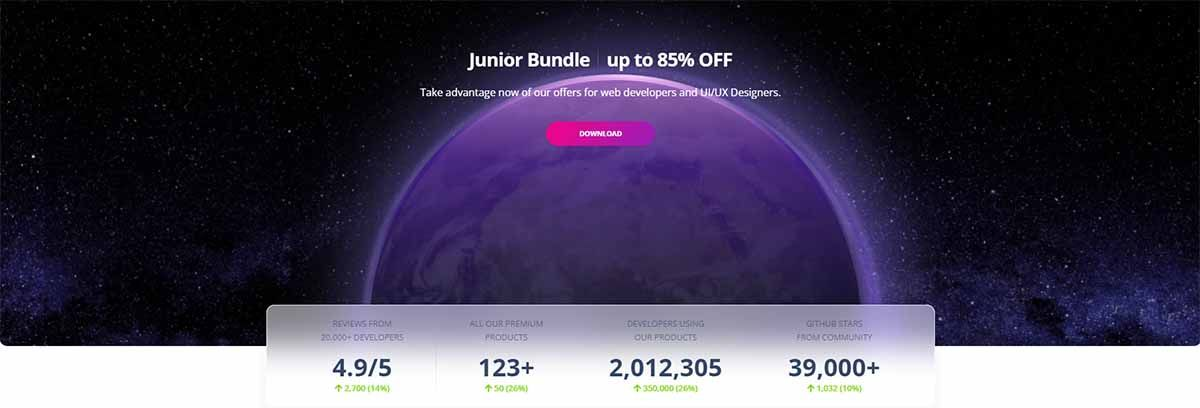
Here are the topics covered in this article:
- ✅ Why do we need forms?
- ✅ Intro to
React
(a short one) - ✅
Formik
: pros, cons, and sample - ✅
React Hook Form
: pros, cons, and sample - ✅
React Final Form
: pros, cons, and sample - ✅ Conclusions & Resources
- ✅ Free
React Sample
: Berry (use Formik) - ✅ Free
React Sample
: Datta Able (use Formik)
✨ Why do we need forms
As mentioned above, forms might seem trivial and easy to code, but this fact is not true in all cases. Forms require validating the information provided by the user and coding multiple forms in different parts of our projects might be time-consuming. To solve this problem, we can use Form Libraries built on top of React
released in the open-source ecosystem like Formik
or React Final Form
.
All mentioned libraries are easy to use and have (almost) all the functionalities we might need in our projects.
All we have to do is install and perform a quick setup of our preferred library. After that, we should be able to code much faster all forms required in the project and spend more time developing the actual business logic for the app.
✨ What is React
React is an open-source UI library, built and maintained by Facebook. React breaks down a big application into smaller pieces called components. This component-based approach of React makes it more usable and maintainable.
As you’ll see React is not a framework it’s a library. That means it’s not a complete solution.
New to React?
Check out this comprehensive React Starting Guide
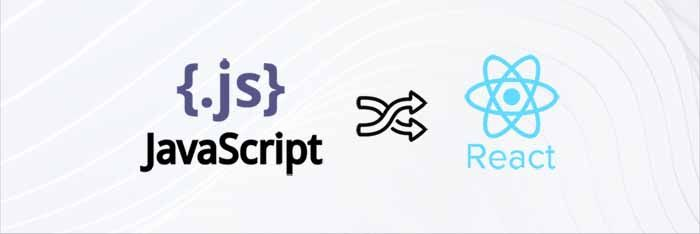
When you build an application with react you’ll need to use other external libraries to finish the application. This approach of React makes it more powerful because you have the freedom to choose whatever library you want for your app.
Examples of the libraries you might need to use are Axios
for HTTP requests, Redux
for managing states, and React-router-dom
for Routing, `React-hook-form` for building forms.
✨ Why choose the right Form Library?
Might be important what form library you choose for your application because some libraries are large and slow, and a lot of boilerplate codes or lack of support. These issues make using the library difficult.
For these reasons you have to be picky about the library you choose for your application. So, without any delay let's dive in and see the pros and cons of the top three React Form Libraries.
We’ll start with Formik.
✨ Formik
Formik
is an open-source React form library Authored by Jared Palmar
. Jared built Formik
out of frustration when he had to build more than 30 forms for his application. He wanted a standard way to build and manage all the forms in his application. For this he built Formik
.
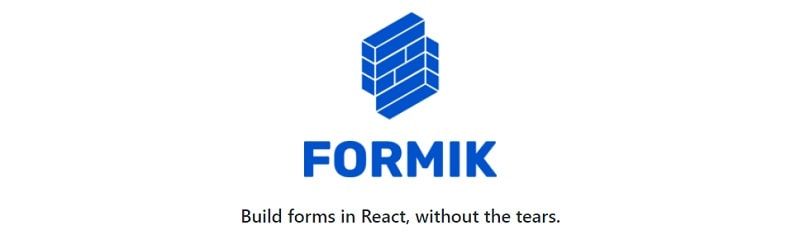
This library helps you with the three most annoying but important parts of building forms:
- 👉 Getting values in and out of form state
- 👉 Validation and error messages
- 👉 Handling form submission
You can build a form easily with Formik’s <Formik />
, <Form />
, <Field />
, and <ErrorMessage />
components. Building a form with Formik is super easy. Later In this section, I’ll show you how to build a form and validate it with Formik.
Before that let’s look at a few pros and cons of Formik.
Formik Pros
- Declarative components (
<Formik />
,<Form />
,<Field />
) - 29k+ stars on Github
- Integration with popular validation library:
Yup
- External
UI Library
support.
Formik Cons
- 7 dependencies and
12.7
kB in size - 500+ Issues on GH
- No built-in validation
- A lot of boilerplate code (compared to React-hook-form)
Now that we’ve seen the pros and cons of Formik
, let’s build a simple form with Formik
to make it clear.
Install Formik
To build a form with Formik first we’ll have to install it. We can do that with these commands:
$ npm install formik
// OR
$ yarn add formik
Form Source Code
import React from 'react';
import ReactDOM from 'react-dom';
import { Formik, Field, Form } from 'formik';
const Basic = () => (
<div>
<h1>Sign Up</h1>
<Formik
initialValues={{
firstName: '',
lastName: '',
email: '',
}}
onSubmit={async (values) => {
await new Promise((r) => setTimeout(r, 500));
alert(JSON.stringify(values, null, 2));
}}
>
<Form>
<label htmlFor="firstName">First Name</label>
<Field id="firstName" name="firstName" placeholder="Jane" />
<label htmlFor="lastName">Last Name</label>
<Field id="lastName" name="lastName" placeholder="Doe" />
<label htmlFor="email">Email</label>
<Field
id="email"
name="email"
placeholder="jane@acme.com"
type="email"
/>
<button type="submit">Submit</button>
</Form>
</Formik>
</div>
);
ReactDOM.render(<Basic />, document.getElementById('root'));
As you can see it’s a simple form. We didn’t add validation yet. I’ll do that in the next section.
Now, let’s look at what’s going on above.
To build the Form we called the <Formik>
component. It takes two props: initialValues
object which defines the initial values of the input fields and onSubmit
method which handles form submission.
As you noticed, this form is self-declarative. That means the code describes what’s going on. you don’t need much effort to understand it.
Once we have finished the basic form, the next step is to add validation and constraint user to input relevant information.
👉 Form Validation in Formik
One of the reasons to build Formik is to make form validation hassle-free. Formik supports synchronous and asynchronous Form-level
and Field-level validation
. It also provides schema-based Form-level validation through Yup
. Yup
is a very popular form validation library.
Form level validation
To add form level validation you need to add the validation props to the <Formik> component and pass your form validation function.
React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
const FormikForm = () => {
return <div>
Formik Form
<Formik
initialValues={{ email: '', password: '' }}
validate={values => {
const errors = {};
if (!values.email) {
errors.email = 'Required';
} else if (
!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}$/i.test(values.email)
) {
errors.email = 'Invalid email address';
}
if (!values.password) {
errors.password = "Required";
} else if (values.password.length < 8) {
errors.password = "Password too short";
}
return errors;
}}
onSubmit={(values, { setSubmitting }) => {
setTimeout(() => {
alert(JSON.stringify(values, null, 2));
setSubmitting(false);
}, 400);
}}
>
{({ isSubmitting }) => (
<Form>
<label>Email</label>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
<label>Password</label>
<Field type="password" name="password" />
<ErrorMessage name="password" component="div" />
<button type="submit" disabled={isSubmitting}>
Submit
</button>
</Form>
)}
</Formik>
</div>
};
export default FormikForm;
This is just the initialization of validation. Now we need a way to display the error message. You can do that easily with the <ErrorMessage>
component like this:
<label>Password</label>
<Field type="password" name="password" />
<ErrorMessage name="password" component="div" />
Make sure you use the same name in the field and ErrorMessage component. You can see the complete code above. Now, let’s look at field-level validation.
Field level validation
Field-level validation
is not that different from Form-level validation
. All you have to do is add your validation form to the Field instead of the Form.
import React from 'react';
import { Formik, Form, Field } from 'formik';
function validateEmail(value) {
let error;
if (!value) {
error = 'Required';
} else if (!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i.test(value)) {
error = 'Invalid email address';
}
return error;
}
function validateUsername(value) {
let error;
if (value === 'admin') {
error = 'Nice try!';
}
return error;
}
export const FieldLevelValidationExample = () => (
<div>
<h1>Signup</h1>
<Formik
initialValues={{
username: '',
email: '',
}}
onSubmit={values => {
// same shape as initial values
console.log(values);
}}
>
{({ errors, touched, isValidating }) => (
<Form>
<Field name="email" validate={validateEmail} />
{errors.email && touched.email && <div>{errors.email}</div>}
<Field name="username" validate={validateUsername} />
{errors.username && touched.username && <div>{errors.username}</div>}
<button type="submit">Submit</button>
</Form>
)}
</Formik>
</div>
);
To add the validation function to the field you need to do a little tweak. You need to wrap the form with an unnamed function, as I did above.
And that function will take arguments such as errors
, touched
, isValidating
.
Also to show the errors you can’t use the ErrorMessage here you’ll need to write it like this:
{errors.email && touched.email && <div>{errors.email}</div>}
This is it. A complete Form built with Formik
. For more resources, feel free to access:
✨ React Hook Form
React Hook Form is a form library built around React hooks. This library takes a different approach to building Form. It isolates component re-renders by using uncontrolled components.
Unlike Formik
, React-Hook-Form
has zero dependencies. And the size is half the size of Formik ~= 8.6KB (minified + gzipped).
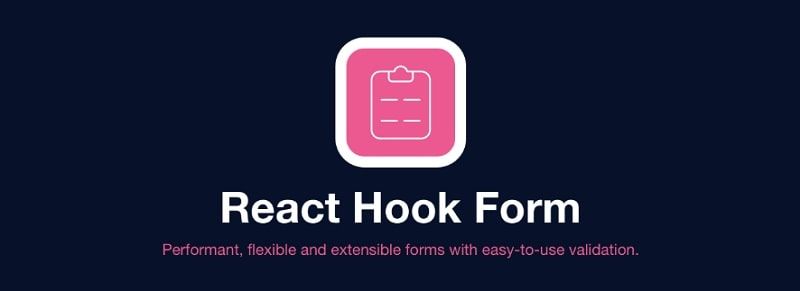
React Hook Form reduces the amount of code you need to write while removing unnecessary re-renders. To validate forms React-hook-form uses the standard Html based approach. You can build a form with React-hook-form easily with the useForm
hook. I’ll show you how to do that later in the post.
But now let’s look at a few Pros and Cons of React Hook Form
.
React Hook Form Pros
- Less boilerplate code, Zero dependency
- No issues on Github (what?)
- 8.6kb size (minified and gzipped)
Out of the box
integration with UI libraries- Embraces native HTML form validation
- Support’s
Yup
form validation.
React Hook Form Cons
- You need to use Yup or Built-in form validation
Install React Hook Form
$ npm install react-hook-form
// OR
$ npm install react-hook-form
Form Source Code
import { useForm } from "react-hook-form";
const HookForm = () => {
const { register, handleSubmit } = useForm();
const handleRegistration = (data) => console.log(data);
return (
<div>
Hook Form
<form onSubmit={handleSubmit(handleRegistration)}>
<div>
<label>Email</label>
<input type="email" name="email" {..register('email')} />
</div>
<div>
<label>Password</label>
<input type="password" name="pass" {..register('pass')} />
</div>
<button>Submit</button>
</form>
</div>
);
};
The handleSubmit
handles the form submission and the register
method helps you register an input field into React Hook Form
so that it is available for validation, and its value can be tracked for changes.
This is a basic form. Now let’s look at how you can validate forms with react-hook-form
:
Form validation in React Hook Form
React Hook Form uses native HTML form validation to validate forms. To validate using you pass the validation rules to the register method.
The validation rules are:
required
: indicates if the field is required or not.minlength
andmaxlength
set the minimum and maximum length for a string input valuemin
andmax
set the minimum and maximum values for a numerical value- pattern: takes a regular expression pattern to test the input.
Here’s an example of how you validate forms in React-hook-form
:
export default function App() {
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {..register("firstName", { required: true, maxLength: 20 })} />
<input {..register("lastName" , { pattern: /^[A-Za-z]+$/i })} />
<input type="number" {..register("age", { min: 18, max: 99 })} />
<input type="submit" />
</form>
);
}
As you noticed this is just the initialization of validation. We’ll need a way to show the error message to the user.
import React from "react";
import { useForm } from "react-hook-form";
export default function App() {
const { register, formState: { errors }, handleSubmit } = useForm();
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input {...register("firstName", { required: true })} />
{errors.firstName?.type === 'required' && "First name is required"}
<input {...register("lastName", { required: true })} />
{errors.lastName && "Last name is required"}
<input type="submit" />
</form>
);
}
For more resources, related to React-hook-form
, feel free to access:
- 👉 https://react-hook-form.com/get-started#Quickstart
- 👉 https://blog.logrocket.com/react-hook-form-complete-guide/
✨ React Final Form
React Final Form
is a thin wrapper around Final Form. It doesn't have any other dependency than the Final Form
and the size is really lightweight ~= 3.3kb
(minified and gzipped).
You can build forms with React Final Form easily using the Form and Field component of React Final Form. I’ll show you how to do that in a few moments.
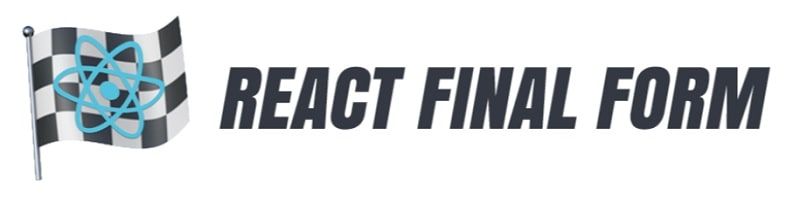
Before we do that, let’s look at the Pros and Cons of the React Final Form.
Pros
- renders only the changed inputs
- only 3.2kb (minified and gzipped)
- 6,884 stars on Github
Cons
- Has a peer dependency:
Final Form
. - A lot of boilerplate code
- 350+ issues on Github
Install React Final Form
$ npm install final-form react-final-form
// OR
$ yarn final-form react-final-form
To build a form you’ll need to import the Form and Field component from react-final-form
.
Form Sample Source Code
import { Form, Field } from 'react-final-form'
import React from 'react'
import { render } from 'react-dom'
import Styles from './Styles'
import { Form, Field } from 'react-final-form'
const sleep = ms => new Promise(resolve => setTimeout(resolve, ms))
const onSubmit = async values => {
await sleep(300)
window.alert(JSON.stringify(values, 0, 2))
}
const App = () => (
<Styles>
<h1>React Final Form - Simple Example</h1>
<Form
onSubmit={onSubmit}
initialValues={{ firstname: '', lastname :''}}
render={({ handleSubmit, form, submitting, pristine, values }) => (
<form onSubmit={handleSubmit}>
<div>
<label>First Name</label>
<Field
name="firstName"
component="input"
type="text"
placeholder="First Name"
/>
</div>
<div>
<label>Last Name</label>
<Field
name="lastName"
component="input"
type="text"
placeholder="Last Name"
/>
</div>
<div className="buttons">
<button type="submit" disabled={submitting || pristine}>
Submit
</button>
<button
type="button"
onClick={form.reset}
disabled={submitting || pristine}
>
Reset
</button>
</div>
</form>
)}
/>
</Styles>
)
render(<App />, document.getElementById('root'))
As you can see this form is almost like the Formik
form. To build a form the <Form/>
component takes 3 props: initialValues
, handleSubmit
and render
.
This is a basic form with React Final Form
without validation. The validation in React Final Form
is provided in two flavors, just like Formik
- 👉 Form-level validation
- 👉 Field-level validation
Because the validation code pattern is quite similar to Formik
we will skip the samples for now. For more information related to React Final Form
, please access:
- 👉 https://final-form.org/docs/react-final-form/api
- 👉 https://blog.logrocket.com/build-high-performance-forms-using-react-final-form/
✨ So, Which one should you choose?
Choosing a form library should be dependent on what type of project you are building. Still, there are a few global points to choosing one library over another.
After all these comparisons If I start any project right now, I’ll choose React-Hook-Form
, because it has zero dependencies and less boilerplate code compared to React Final Form
and Formik
.
Formik
and React-Final-Form
make forms complicated while React-Hook-Form
looks cleaner and more intuitive at first look. Also, the validation provided by React-Hook-Form
uses less code to finish the job.
At the end of the day, feel free to choose Formik
, or React-Final-Form
for your project. It’s completely up to your personal preference.
Sorry for this super long article. For those that are still here, the next section presents a few React Products that use production-ready React Forms
.
✨ React Berry (uses Formik
)
Berry is a creative React Dashboard built using the Material-UI. It is meant to be the best User Experience with highly customizable feature-riched pages. It is a complete game-changer React Dashboard with an easy and intuitive responsive design on retina screens or laptops.
- 👉 React Node JS Berry - product page
- 👉 React Node JS Berry - LIVE Demo
The product comes with a simple JWT authentication flow: login
/register
/logout
powered by an open-source Node JS API Backend via Passport
Library.
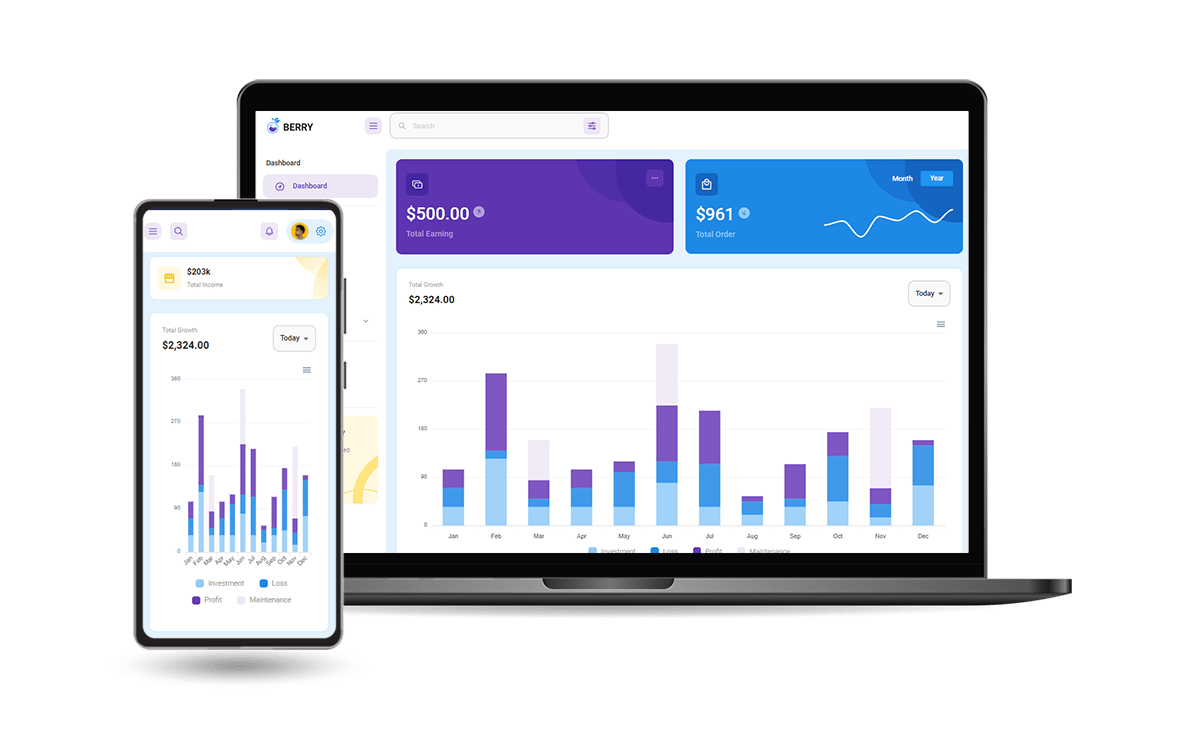
✨ React Datta Able - (uses Formik
)
Datta Able is an open-source React Dashboard that provides a colorful and modern design. Datta Able React Free is the most stylized React Free Admin Template, around all other admin templates in the market.
- 👉 React Node Datta Able - product page
- 👉 React Node Datta Able - LIVE Demo
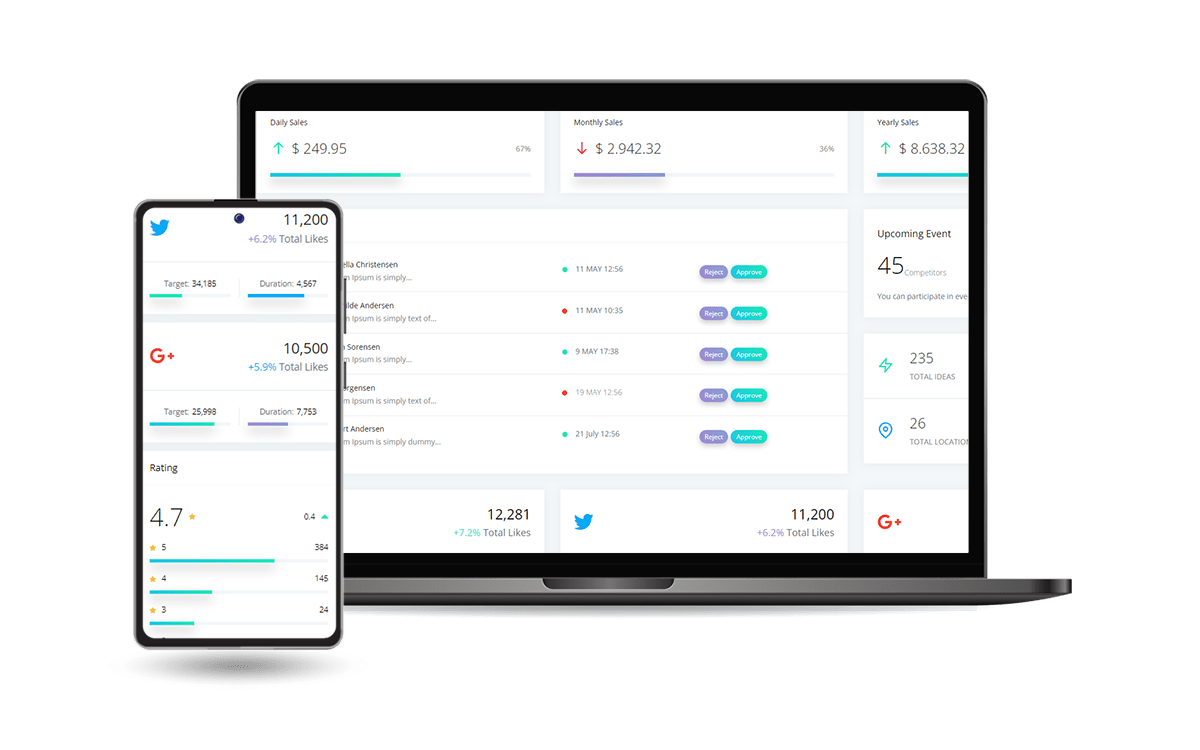