Simple Flask API Server
Open-source API server that might help beginners to understand better the API concept
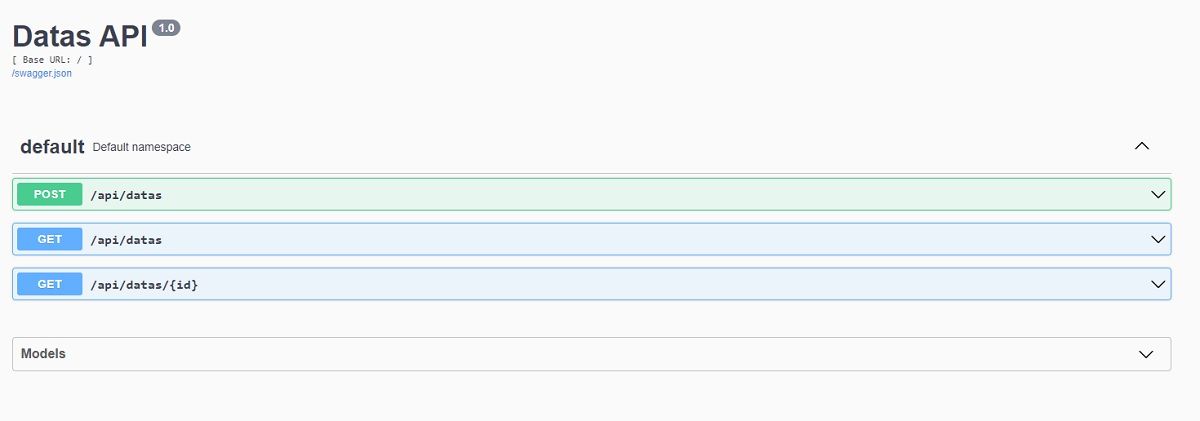
Hello! This article presents a simple API starter that might help beginners to understand better the API concept. The codebase can be downloaded from Github and used for eLearning activities or production. The framework that powers the API is Flask, a leading software library actively supported and versioned by many open-source
enthusiasts. Thanks for reading!
- 👉 Flask API Sample - source code
- 🎁 Free support via email and Discord (1k+ community).
✨ Starter Features
- 👉 Simple API over a minimal
Datas
table - 👉
SQLite Persistence
managed by an elegant ORM (SqlAlchemy) - 👉
Powerful API
core provided by Flask-RestX - 👉
Strong Input validation
API Definition
Route | Verb | Info | Status |
---|---|---|---|
/datas |
GET | return all items | ✔️ |
POST | create a new item | ✔️ | |
/datas:id |
GET | return one item | ✔️ |
PUT | update item | ✔️ | |
DELETE | delete item | ✔️ |
✨ API Coding & Implementation Rules
- Simple Interface
- Consistent, intuitive actions
- Strong Input Validation
✨ Codebase Structure
All relevant files are listed below. Other files like docker-compose.yml
, README, LICENSE are omitted.
api-server-flask/
├── api
│ ├── __init__.py
│ ├── config.py
│ ├── models.py
│ └── routes.py
├── README.md
├── requirements.txt
└── run.py
✨ API Models
The information managed by the API is saved using a simple table defined with three fields: id
, data
, date_created
. Here is the source code:
# Contents of "api/models.py" (truncated)
...
class Datas(db.Model):
id = db.Column(db.Integer() , primary_key=True)
data = db.Column(db.String(256) , nullable=False)
date_created = db.Column(db.DateTime() , default=datetime.utcnow)
...
The source code provides a few helpers that make our life, as a developer, easier:
update_data
- update thedata
fieldsave
- save & commit the updates of the current objecttoJSON
- returns the JSON representation
✨ Routing
Each method is kept as simple as possible but at the same time, provides robust validation and elegant SQL access.
For instance, the route that manages the update operation for an item
:
# Contents of "api/routes.py" (truncated)
....
@rest_api.route('/api/datas/<int:id>')
class ItemManager(Resource):
...
"""
Update Item
"""
@rest_api.expect(update_model, validate=True)
def put(self, id):
item = Datas.get_by_id(id)
# Read ALL input from body
req_data = request.get_json()
# Get the information
item_data = req_data.get("data")
if not item:
return {"success": False,
"msg": "Item not found."}, 400
item.update_data(item_data)
item.save()
return {"success" : True,
"msg" : "Item [" +str(id)+ "] successfully updated",
"data" : item.toJSON()}, 200
...
Let's iterate over the relevant lines:
@rest_api.route('/api/datas/<int:id>')
defines the route
Flask will route the request to this section when users access /api/datas/1
for instance.
@rest_api.expect(update_model, validate=True)
This decorator triggers a validation previously defined as bellow:
update_model = rest_api.model('UpdateModel', {"data": fields.String(required=True, min_length=1, max_length=255)})
If the data
field has a size over 255, the request is rejected. For us, as developers, the coding effort is minimal.
✨ Where to go from here
This simple API will be extended with more features soon:
- Add more fields to
Datas
model - Implement authentication
- restrict
update
actions to authenticated users.
For more resources, feel free to access:
- 👉 Flask - the official website
- 👉 Flask-RestX - library docs
- 👉 More Flask Starters provided by AppSeed