Python - How "Import" Directive Works
Learn how to use the "import" directive works in Python, a leading language for programmers - provided by AppSeed
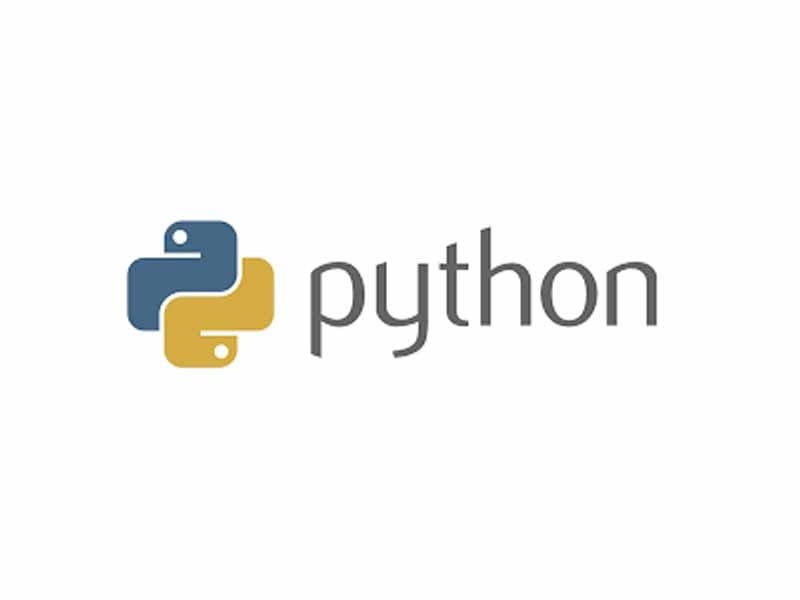
Hello Coders! This article explains the "import" directive in Python. How it works and how can be used in different contexts. Thanks for reading!
The import
directive in Python is used to bring modules or packages into your current namespace, allowing you to use the functionality defined within them. Let's break down how it works in detail:
Basic Importing:
You can import a module or package in Python using the import
keyword followed by the name of the module or package:
import module_name
or
import package_name
Using Imported Items:
Once imported, you can use functions, classes, or variables defined in the module or package by prefixing them with the module or package name:
module_name.function_name()
or
package_name.module_name.function_name()
Importing Specific Items:
You can import specific items from a module instead of the entire module using the from
keyword:
from module_name import function_name
You can also import multiple items from a module using comma separation:
from module_name import function_name1, function_name2
Aliasing:
You can provide an alias to imported modules or items using the as
keyword:
import module_name as alias_name
or
from module_name import function_name as alias_name
Importing All Items:
You can import all items from a module using the *
wildcard:
from module_name import *
However, it's generally not recommended because it can lead to namespace pollution and makes it unclear where each item is coming from, and also imports items unused in the current context.
Importing Packages:
If you want to import a module from a package, you use dot notation:
import package_name.module_name
or
from package_name import module_name
Execution of Import Statements:
Import statements are executed just like any other statements, and they are typically executed only once per interpreter session. After a module is imported, it's cached in sys.modules
, so subsequent imports of the same module do not cause it to be re-executed.
Conclusion:
In summary, the import
directive in Python is a powerful tool for bringing in functionality from modules and packages. It allows you to organize your code into reusable and modular components, making it easier to manage and maintain large codebases. Understanding how to use import
effectively is essential for writing clean and maintainable Python code.
Thanks for reading! For more resources, feel free to access:
- 👉 AppSeed, a platform already used by 8k registered developers
- 👉 Build apps with Django App Generator (free service)